|
| 1 | +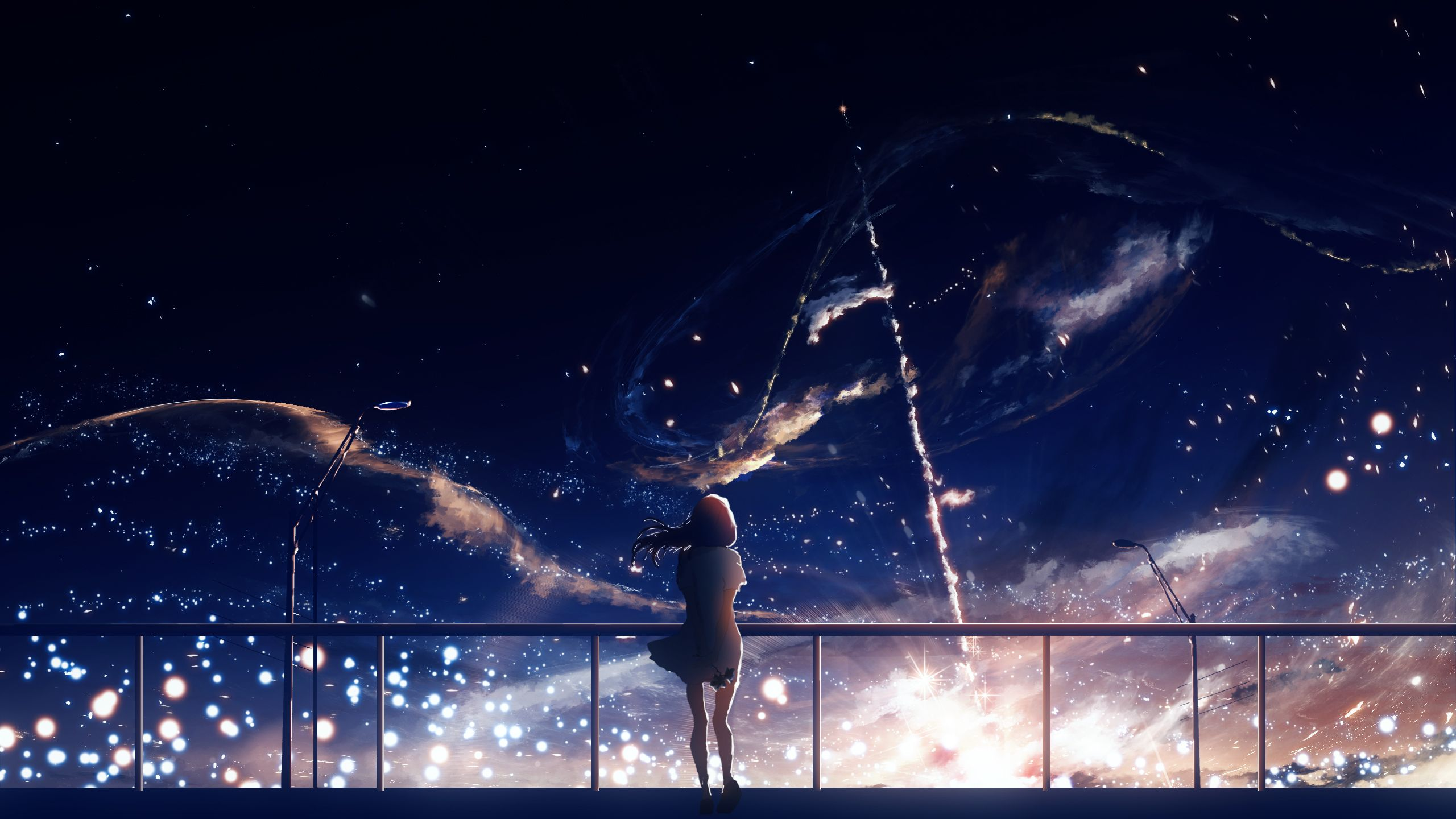 |
| 2 | +>仰望星空的人,不应该被嘲笑 |
| 3 | +
|
| 4 | +## 题目描述 |
| 5 | + |
| 6 | +反转从位置 m 到 n 的链表。请使用一趟扫描完成反转。 |
| 7 | + |
| 8 | +说明: |
| 9 | +1 ≤ m ≤ n ≤ 链表长度。 |
| 10 | + |
| 11 | +示例: |
| 12 | + |
| 13 | +```javascript |
| 14 | +输入: 1->2->3->4->5->NULL, m = 2, n = 4 |
| 15 | +输出: 1->4->3->2->5->NULL |
| 16 | +``` |
| 17 | + |
| 18 | +来源:力扣(LeetCode) |
| 19 | +链接:https://leetcode-cn.com/problems/reverse-linked-list-ii |
| 20 | +著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。 |
| 21 | + |
| 22 | + |
| 23 | +## 解题思路 |
| 24 | + |
| 25 | +**借助递归** |
| 26 | + |
| 27 | +```javascript |
| 28 | +/** |
| 29 | + * Definition for singly-linked list. |
| 30 | + * function ListNode(val) { |
| 31 | + * this.val = val; |
| 32 | + * this.next = null; |
| 33 | + * } |
| 34 | + */ |
| 35 | +/** |
| 36 | + * @param {ListNode} head |
| 37 | + * @param {number} m |
| 38 | + * @param {number} n |
| 39 | + * @return {ListNode} |
| 40 | + */ |
| 41 | +var reverseBetween = function (head, m, n) { |
| 42 | + let reverse = (pre, cur) => { |
| 43 | + if (!cur) return pre; |
| 44 | + let tmp = cur.next; |
| 45 | + cur.next = pre; |
| 46 | + return reverse(cur, tmp); |
| 47 | + } |
| 48 | + let dummyHead = new ListNode(); |
| 49 | + dummyHead.next = head; |
| 50 | + let p = dummyHead; |
| 51 | + let k = m - 1; |
| 52 | + // 先找到需要反转链表部分的前驱节点 |
| 53 | + while (k--) { |
| 54 | + p = p.next; |
| 55 | + } |
| 56 | + // 保存前驱节点 |
| 57 | + let front = p; |
| 58 | + // 找到需要反转链表部分的头节点 |
| 59 | + let frontNode = front.next; |
| 60 | + k = n - m + 1; |
| 61 | + // 再找到需要反转链表部分的尾节点 |
| 62 | + while (k--) { |
| 63 | + p = p.next; |
| 64 | + } |
| 65 | + // 找到需要反转链表部分的尾节点 |
| 66 | + let endNode = p; |
| 67 | + // 保存后继节点 |
| 68 | + let end = endNode.next; |
| 69 | + // 将后继值为空,开始反转链表 |
| 70 | + endNode.next = null; |
| 71 | + front.next = reverse(null, frontNode); |
| 72 | + // 原本的反转链表部分的头节点现在变成了尾节点,指向原本的后继节点 |
| 73 | + frontNode.next = end; |
| 74 | + return dummyHead.next; |
| 75 | +}; |
| 76 | +``` |
| 77 | + |
| 78 | +**迭代解法** |
| 79 | + |
| 80 | +```javascript |
| 81 | +/** |
| 82 | + * Definition for singly-linked list. |
| 83 | + * function ListNode(val) { |
| 84 | + * this.val = val; |
| 85 | + * this.next = null; |
| 86 | + * } |
| 87 | + */ |
| 88 | +/** |
| 89 | + * @param {ListNode} head |
| 90 | + * @param {number} m |
| 91 | + * @param {number} n |
| 92 | + * @return {ListNode} |
| 93 | + */ |
| 94 | +var reverseBetween = function(head, m, n) { |
| 95 | + let dummyHead = new ListNode(); |
| 96 | + dummyHead.next = head; |
| 97 | + let p = dummyHead; |
| 98 | + let k = m-1; |
| 99 | + // 先找到需要反转链表部分的前驱节点 |
| 100 | + while (k--) { |
| 101 | + p = p.next; |
| 102 | + } |
| 103 | + // 保存前驱节点 |
| 104 | + let front = p; |
| 105 | + let pre = frontNode = front.next; |
| 106 | + let cur = pre.next; |
| 107 | + k = n-m; |
| 108 | + // 长度为3的链表需要反转2次,那么长度为n的链表需要反转n-1次 |
| 109 | + while(k--){ |
| 110 | + let tmp = cur.next; |
| 111 | + cur.next = pre; |
| 112 | + pre = cur; |
| 113 | + cur = tmp; |
| 114 | + } |
| 115 | + // 将原本前驱节点的next指向当前反转后的链表 |
| 116 | + front.next = pre; |
| 117 | + // 原本反转链表的头节点现在变成了尾结点,指向后继节点 |
| 118 | + frontNode.next = cur; |
| 119 | + return dummyHead.next; |
| 120 | +}; |
| 121 | +``` |
| 122 | + |
| 123 | +## 最后 |
| 124 | +文章产出不易,还望各位小伙伴们支持一波! |
| 125 | + |
| 126 | +往期精选: |
| 127 | + |
| 128 | +<a href="https://github.com/Chocolate1999/Front-end-learning-to-organize-notes">小狮子前端の笔记仓库</a> |
| 129 | + |
| 130 | +<a href="https://github.com/Chocolate1999/leetcode-javascript">leetcode-javascript:LeetCode 力扣的 JavaScript 解题仓库,前端刷题路线(思维导图)</a> |
| 131 | + |
| 132 | +小伙伴们可以在Issues中提交自己的解题代码,🤝 欢迎Contributing,可打卡刷题,Give a ⭐️ if this project helped you! |
| 133 | + |
| 134 | + |
| 135 | +<a href="https://yangchaoyi.vip/">访问超逸の博客</a>,方便小伙伴阅读玩耍~ |
| 136 | + |
| 137 | +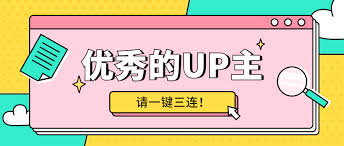 |
| 138 | + |
| 139 | +```javascript |
| 140 | +学如逆水行舟,不进则退 |
| 141 | +``` |
| 142 | + |
| 143 | + |
| 144 | + |
0 commit comments