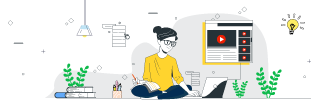
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
3Sum Problem in Python
Suppose we have an array of numbers. It stores n integers, there are there elements a, b, c in the array, such that a + b + c = 0. Find all unique triplets in the array which satisfies the situation. So if the array is like [-1,0,1,2,-1,-4], then the result will be [[-1, 1, 0], [-1, -1, 2]]
To solve this, we will follow these steps −
- Sort the array nums, and define an array res
- for i in range 0 to length of nums – 3
- if i > 0 and nums[i] = nums[i - 1], then skip the next part and continue
- l := i + 1 and r := length of nums – 1
- while l < r
- sum := sum of nums[i], nums[l] and nums[r]
- if sum < 0, then l := l + 1, otherwise when sum > 0, then r := r – 1
- otherwise insert nums[i], nums[l], nums[r] into the res array
- while l < length of nums – 1 and nums[l] = nums[l + 1]
- increase l by 1
- while r > 0 and nums[r] = nums[r - 1]
- decrease r by 1
- increase l by 1 and decrease r by 1
- return res
Example(Python)
Let us see the following implementation to get better understanding −
class Solution(object): def threeSum(self, nums): nums.sort() result = [] for i in range(len(nums)-2): if i> 0 and nums[i] == nums[i-1]: continue l = i+1 r = len(nums)-1 while(l<r): sum = nums[i] + nums[l] + nums[r] if sum<0: l+=1 elif sum >0: r-=1 else: result.append([nums[i],nums[l],nums[r]]) while l<len(nums)-1 and nums[l] == nums[l + 1] : l += 1 while r>0 and nums[r] == nums[r - 1]: r -= 1 l+=1 r-=1 return result ob1 = Solution() print(ob1.threeSum([-1,0,1,2,-1,-4]))
Input
[-1,0,1,2,-1,-4]
Output
[[-1,-1,2],[-1,0,1]]
Advertisements