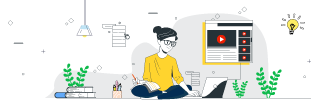
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Clear Regular Expression Cache in Python
This article talks about how you can clear the regular expression cache in Python. Regular expressions in Python are used to find patterns in text, but they also use an internal cache to store frequently used expressions for faster execution.
We may sometimes need to clear this cache to free up memory or reset stored patterns. You can do this using Python's re.purge() method.
Why Clear Regex Cache?
You need to clear the regex cache for the following reasons -
Too many stored patterns may consume your system's memory.
If you are dynamically updating regex patterns, deleting the cache memory makes sure the prior patterns do not conflict.
When working with short-lived regex operations, avoid maintaining unnecessary cache.
How to Clear the Regex Cache?
To clear the regex cache, you can use the re.purge() method to remove all the stored regex patterns. It is a very simple function that does not accept any parameters. Here you can see the simple usage of re.purge() function -
# Import the re module import re # Clear regex cache re.purge() # Print the message print("Regex cache cleared!")
Output
This will create the following outcome -
Regex cache cleared!
Now we will see different examples to see how you can use re.purge() function to clear the regex cache in Python.
Example 1
The following example will show how the cache works by using a pattern before clearing it using the re.purge() function. Here we are using the "\d{3}-\d{2}-\d{4}" pattern to match a number format. So our program basically stores a pattern in cache, clears it, and confirms the removal.
# Import the re module import re # Compile a regex pattern pat = re.compile(r"\d{3}-\d{2}-\d{4}") # Clear cache re.purge() # Print the message print("Cache cleared, pattern removed!")
Output
This will generate the following output -
Cache cleared, pattern removed!
Example 2
Now we are creating a function to clear the cache so that we can make it reusable. So it basically making a functionality for cache clearing in a reusable function.
# Import the re module import re # Create a function to clear cache def clear_cache(): re.purge() print("Regex cache reset!") # Call the function clear_cache()
Output
This will generate the following result -
Regex cache reset!
Example 3
In the example below, we are going to store multiple regex patterns. After storing them, we will clear the cache and print a confirmation message to the console.
# Import the re module import re # Compile multiple patterns p1 = re.compile(r"\d+") p2 = re.compile(r"[A-Za-z]+") # Clear cache re.purge() # Print the message print("All stored regex patterns cleared!")
Output
This will produce the following result -
All stored regex patterns cleared!