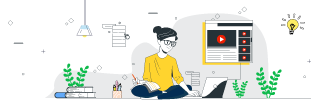
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Concatenate a String with Numbers in Python
Concatenation is nothing but the process of joining two or more strings together. In Python, if one value is a number (integer or float), we can't directly concatenate it with a string using the '+' operator, as it raises a TypeError.
Python does not allow the implicit conversion between strings and numbers during the concatenation. So, we need to explicitly convert the numbers to a string.
Using Python str() Function
The Python str() function is used to convert the given value into a string. In this approach, we are going to apply the str() function to the integer to convert it to a string and then join the two strings using the '+' operator.
Syntax
Following is the syntax for the Python str() function -
str(x)
Example
Let's look at the following example, where we are going to use the str() function for concatenating a string with a number.
name = "Tutorialspoint_" year = 2025 result = name + str(year) print(result)
The output of the above program is as follows -
Tutorialspoint_2025
Using Python format() Method
The Python format() method is used to format the string by replacing the placeholders with the corresponding values.
Syntax
Following is the syntax for the Python format() method -
str.format(value1, value2...)
Example
Consider the following example, where we are going to use the format() method to concatenate the string with numbers.
num = 6 result = "You have {} notifications".format(num) print(result)
The following is the output of the above program -
You have 6 notifications
Using Python f-strings
The third approach is by using the f-strings. In Python, f-strings are the efficient way to format strings. They are created by prefixing a string with the letter f or F. Inside the string, expressions or variables can be placed within the curly braces {}.
The python f-stings are introduced in the python 3.6, before that we had to use the format() method.
Example
In the following example, we are going to use the f-strimgs and observe the output.
x = "Welcome_To_" y = 2025 print("The concatenated string is : ") result = f'{x}{y}' print(result)
The output of the above program is as follows -
The concatenated string is : Welcome_To_2025
Using % Operator
The fourth approach is by using %, we should write %s for each integer and string inside the quotes, as we want the final output as a string, then we will add the name of the number and string we are using with a %.
Example
Following is an example where we are going to use the "%" operator and concatenate the string with numbers.
a = "Chevrolet_Cheron_" b = 2025 print("The concatenated string is : ") result = '%s%s' % (a, b) print(result)
The output of the above program is as follows -
Chevrolet_Cheron_2025