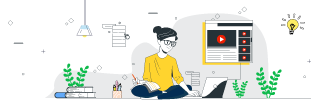
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sort a String with a Number Inside in Python
Sorting the string that contains the number, such as ("xy1", "xy2", "xy10"), can be complex in Python. For example, if we sort the list ["xy1", "xy2", "xy10"] using the built-in sort() method, it results in ["xy1", "xy10", "xy2"]. But we will expect "xy2" to come before "xy10".
It is because the Python default sorting uses the lexicographical order and compares the characters from left to right based on their Unicode values. Since the character '1' in "xy10" comes before "2" in "xy2", the "xy10" is treated as smaller, even though the number 10 is greater than 2.
This is where we need the natural sorting, which understands that "xy2" should come before the "xy10" as it treats the embedded numbers as integers instead of strings. For implementing this kind of sorting, we can use regular expressions. In this article, we are going to explore the different ways to sort the strings with numbers inside using Python.
Using Python re.split() Method
The first approach is by using the Python re.split() method, It is used to split the string by the occurrences of a specified regular expression.
Here, we are going to use the regex pattern '(\d+)' along with re.split() method to split the strings into parts, separating digits and non-digits. We then convert the digit parts to integers so that sorting behaves numerically.
Syntax
Following is the syntax of Python re.split() method -
re.split(pattern, string)
Example
Let's look at the following example, where we are going to sort the list ["xy1", "xy10", "xy2"] using the re module.
import re def demo(s): return [int(x) if x.isdigit() else x.lower() for x in re.split(r'(\d+)', s)] str1 = ["xy1", "xy10", "xy2"] str1.sort(key=demo) print(str1)
The output of the above program is as follows -
['xy1', 'xy2', 'xy10']
Using Python sorted() Function
In this approach, we are using the Python sorted() function, which returns a new sorted list from the characters in an iterable object. The order of sorting can be set to either ascending or descending order. However, strings are sorted alphabetically and numbers are sorted numerically.
Syntax
Following is the syntax of the Python sorted() function -
sorted(iterObject, key, reverse)
Example
Consider the following example, where we are going to use the sorted() function to sort without changing the original list.
import re def x(s): return [int(a) if a.isdigit() else a.lower() for a in re.split(r'(\d+)', s)] str1 = ["A1", "A10", "A2"] result = sorted(str1, key=x) print(result)
The output of the above program is as follows -
['A1', 'A2', 'A10']