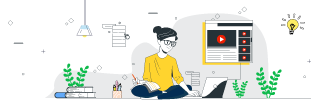
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Declare a Static String Array in Java
In this article, we will learn the declaration of static Strings array in Java. Arrays can be used to store multiple values in one variable. Static array has a specific size which cannot be increased in the later part of the program after creation.
What is a Static String Array?
A static array is a declared array as static, which means that it is associated with the class, not with a class instance. This brings the array into a shared state among all instances of the class. Static variables are loaded when the class is loaded, even before class instances are loaded.
static { array = new String[2]; array[0] = "Hello"; array[1] = "World"; }
A String array is an array which can store a number of String objects. One element in the array can be a string value, and you can access the individual element through an index.
Example
Below is an example of declaring a static string array in Java ?
public class Tester { private static String[] array; static { array = new String[2]; array[0] = "Hello"; array[1] = "World"; } public static void main(String[] args) { System.out.println("Array: "); for(int i = 0; i < array.length; i++){ System.out.print(array[i] + " "); } } }
Output
Array: Hello World
Time complexity: O(n), where n is the size of the array.
Space complexity: O(n), where n is the number of elements in the array.
Why Use a Static Block?
We are using a static block to initialize the static array due to the following reason rather than directly initializing it in the declaration ?
-
Complex Initialization: In case the array needs some logic or computation before it is initialized, we can perform that within a static block.
- Separation of Declaration and Initialization: At times, we might wish to declare the static array but initialize it afterwards in a controlled fashion.
Conclusion
In this article, we explored how to declare and initialize a static String array in Java using a static block. With this method, the array is initialized once upon class loading so that it becomes available for use in all instances of the class.