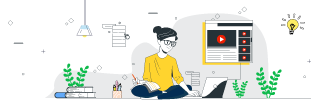
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Open Binary File in Read and Write Mode with Python
Binary file is a file that consists of a series of 1's and 0's. This is typically used to represent data such as images, audio, video, etc. To open the binary files in read and write mode, Python provides an in-built function, which is the open() function.
The open() Function
The Python open() function is a built-in function that is used to open a file. This method accepts a string value representing a file path (or, name) as a parameter and returns the object of the specified file.
In addition to the file path, we can also pass another parameter named mode. This is used to specify the mode of the file in which we need to open it.
Syntax
The syntax for open() function is as follows -
open(file, mode)
Opening a Binary File in Read and Write Mode
To open a binary file in read and write mode, we will use the open() function in the modes 'rb+' and 'wb+'. Following is the description of the two modes -
- rb+ : This mode opens a file in both reading and writing in binary format, and doesn't clear the contents. It requires a file to exist or will return FileNotFoundError.
- wb+ : This mode opens the file in both reading and writing mode in binary format. It doesn't require the file to exist, as it creates a new one if the file doesn't exist. The file is cleared to zero length.
Note: You can use 'rb+' mode to read and modify an existing binary file and use 'wb+' mode to start fresh.
Example
In the example, we will use the 'wb+' mode to open a binary file and clear all the existing content if the file exists, and create a new file if the file doesn't exist.
Further, it writes new content. We will also use the 'rb+' mode to read the file -
# Using wb+:
with open('main.bin', 'wb+') as file:
file.write(b'Welcome to Tutorialspoint!!')
# Using rb+:
with open('main.bin', 'rb+') as f:
print(f.read())
The above code returns the following output -
b'Welcome to Tutorialspoint!!'
Note: The 'b' in the output indicates that the returned string is a byte string, which is a sequence of bytes but not Unicode characters.