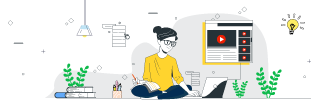
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Correct Syntax to Create Python Dictionary
A Dictionary is a set of key-value pairs, and each key in a Dictionary is separated from its value by a colon ":"; the items are separated by commas. They do not allow duplicate values. Since the 3.7 Python update, dictionaries are ordered.
The correct syntax to create a Python Dictionary is to store values in the form of key-value pairs within the curly braces "{ }". On the left of the colon, we store keys, and on the right, values. The keys should be unique (within one dictionary), and we need to separate the items using commas.
Basic Syntax of the Dictionary
Following is the basic syntax to create a dictionary in Python -
my_dict = { "key1": "value1", "key2": "value2", "key3": "value3" }
Create a Dictionary in Python with 4 key-value pairs
We will create 4 key-value pairs, with keys Product, Model, Units and Available and values Mobile, XUT, 120 and Yes. Keys are on the left of the colon, whereas values are on the right -
Example
# Creating a Dictionary with 4 key-value pairs myprod = { "Product":"Mobile", "Model": "XUT", "Units": 120, "Available": "Yes" } # Displaying the Dictionary print("Dictionary = \n",myprod)
Following is the output of the above program -
Dictionary = {'Product': 'Mobile', 'Model': 'XUT', 'Units': 120, 'Available': 'Yes'}
Create a Dictionary in Python with 5 key-value pairs
We will create 5 key-value pairs, with keys Product, Model, Units, Available, Grades and values Mobile, XUT, 120, Yes, "A" -
Example
# Creating a Dictionary with 5 key-value pairs myprod = { "Product":"Mobile", "Model": "XUT", "Units": 120, "Available": "Yes", "Grades": "A" } # Displaying the Dictionary print("Dictionary = ",myprod)
Following is the output of the above program -
Dictionary = {'Product': 'Mobile', 'Model': 'XUT', 'Units': 120, 'Available': 'Yes', 'Grades': 'A'}
Dictionary with String Keys
A dictionary with string keys is the most common form, where each key is a text label and the value can be any valid Python object. Following is the example which creates a dictionary with keys of string datatype -
person = { "name": "Niharikaa", "age": 30, "city": "New York" "Company:" "Tutorialspoint" } print(type(person)) print("Dictionary = ", person)
Following is the output of the above program -
<class 'dict'> Dictionary = {'name': 'Niharikaa', 'age': 30, 'city': 'New YorkCompany:Tutorialspoint'}
Dictionary with Integer Keys
Python uses integer keys as well, which can be useful for representing mappings based on numbers. Following is an example of creating a dictionary with Integer Keys -
marks = { 101: "Math", 102: "Science", 103: "History" } print(type(marks)) print("Dictionary = ", marks)
Here is the output of the above program -
<class 'dict'> Dictionary = {101: 'Math', 102: 'Science', 103: 'History'}
Mixed Data Type Dictionary
A mixed data type dictionary creates a dictionary with different data types of keys and values. Python dictionaries are highly flexible in this scenario. Following is an example of creating a dictionary with mixed data types -
mixed_dict = { "name": "Niharikaa", 1: [10, 20, 30], "is_active": True } print(type(mixed_dict)) print("Dictionary = ", mixed_dict)
Below is the output of the above program -
<class 'dict'> Dictionary = {'name': 'Niharikaa', 1: [10, 20, 30], 'is_active': True}
Creating an Empty Dictionary
An empty dictionary in Python is created using a pair of curly braces with nothing inside. It can be assigned keys and values later during execution. Following is an example that creates an empty dictionary -
empty_dict = {} print(type(empty_dict)) print("Dictionary = ", empty_dict)
Following is the output of the above program -
<class 'dict'> Dictionary = {}
Using the dict() function
In Python, we have the dict() function, which is used to create a dictionary with defined key and value pairs. This function is particularly useful when creating dictionaries from a sequence of key-value pairs or keyword arguments.
Example
Following is an example of using the dict() function to create a dictionary -
employee = dict(name="Riyaansh", age=28, department="Python") print(employee) data = dict([(1, "one"), (2, "two")]) print(data)
Following is the output of the above program -
{'name': 'Riyaansh', 'age': 28, 'department': 'Python'} {1: 'one', 2: 'two'}