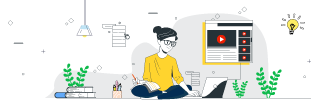
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Pass an Object with a Custom Exception in Python
In Python, you can create your own custom exception classes to represent specific types of errors in your program.
When you raise these custom exceptions, you can also pass an object (like a string or other data) to explain more about what went wrong. This helps make your error messages more useful and detailed.
Creating a Custom Exception Class
In Python, you can pass an object or any extra information with a custom exception by defining a class that inherits from the built-in Exception class.
Inside the custom class, you override the __init__() method to accept additional arguments, and optionally override the __str__() or __repr__() method for custom error messages.
Example
In this example, we are creating a MyCustomError exception that takes a message string and stores it as an instance variable -
class MyCustomError(Exception): def __init__(self, message): self.message = message super().__init__(message) try: raise MyCustomError("Something went wrong!") except MyCustomError as e: print("Caught custom exception:", e.message)
The output will be -
Caught custom exception: Something went wrong!
Passing Custom Objects with Exceptions
You are not just limited to strings, you can also pass entire objects, like dictionaries or custom classes, to provide structured data with your exception.
Example
In this example, we are passing a dictionary object with detailed error information to the custom exception -
class DataError(Exception): def __init__(self, data): self.data = data super().__init__(str(data)) try: error_info = {"code": 400, "message": "Bad input", "field": "username"} raise DataError(error_info) except DataError as e: print("Error details:", e.data)
We get the following output -
Error details: {'code': 400, 'message': 'Bad input', 'field': 'username'}
Accessing Custom Object Attributes
When passing a custom object to your exception, you can store it and access its attributes later to provide specific error-handling behavior or logging.
Example: Passing a user-defined object to a custom exception
In the following example, we are creating a User object and passing it to a custom exception class -
class User: def __init__(self, username): self.username = username class UserError(Exception): def __init__(self, user): self.user = user super().__init__(f"Error with user: {user.username}") try: user = User("suresh_raina") raise UserError(user) except UserError as e: print("Exception for user:", e.user.username)
We get the following output -
Exception for user: suresh_raina
Best Practices
When you pass objects to your custom exceptions in Python, follow these -
- Always call super().__init__() so that the base exception class is initialized correctly.
- Use meaningful names for any attributes you add to store the passed object.
- Make sure your error messages are easy to understand by adding a helpful __str__() method or setting a useful message in the constructor. This makes debugging easier.