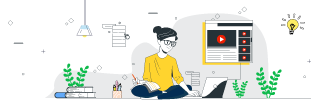
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Use of Return Statement in Python
The return statement in Python is used to return a value or a set of values from a function. When a return statement is encountered in a function, the function execution is stopped, and the value specified in the return statement is returned to the caller.
Without it, a function simply executes its code but doesn't provide any result back. The return statement allows a function to output a result which can be used later in the program.
Why Use the Return Statement?
You should use the return statement in the following cases ?
- To return a result: Use return when you want to return a value or result from a function back to the caller.
- To control function flow: Return can be used to exit a function early if a specific condition is met.
- To improve modularity: Functions with return statements are easier to test and reuse in other parts of the program.
Using the Return Statement in Python
The return statement is used when you need to send a result from a function back to the caller. The function will stop executing once the return statement is encountered and will return the specified value.
Example
Here is an example where the return statement is used to return the sum of two numbers -
# Function using return statement def add_numbers(a, b): return a + b result = add_numbers(5, 3) print(result)
In this example, the function add_numbers() returns the sum of a and b, and the result is printed as follows -
8
Return Statement with No Value
In Python, a function can also use the return statement without specifying a value. This causes the function to return None by default.
Example
In this example, the function will return nothing, so if you print the result of this function, None will be printed -
# Function with return but no value def no_return(): print("This function doesn't return anything.") return result = no_return() print(result)
The output of the above code is as follows -
This function doesn't return anything. None
Using Return with Conditional Statements
The return statement is usually used inside conditional statements. This allows the function to return different results based on certain conditions.
Example
In the following example, the function check_odd_even returns whether a number is odd or even -
def check_odd_even(number): if number % 2 == 0: return "Even" else: return "Odd" result = check_odd_even(4) print(result)
Here, the function checks if the number is divisible by 2. If it is, it returns "Even", otherwise "Odd". The output is -
Even
Returning Multiple Values
In Python, you can also use the return statement to return multiple values from a function. These values are returned as a tuple, which can be unpacked later.
Example
Here is how you can return multiple values from a function -
def get_min_max(numbers): return min(numbers), max(numbers) min_value, max_value = get_min_max([2, 3, 1, 7, 4]) print("Min:", min_value) print("Max:", max_value)
The function get_min_max returns both the minimum and maximum values of a list. The output is -
Min: 1 Max: 7