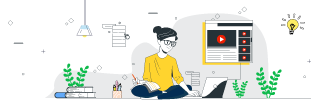
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Python Regular Expression Syntax Explained Simply
This article explains how to work with Regular Expressions (RegEx) in Python using the "re" module. RegEx is a sequence of characters that defines a search pattern.
Below are different ways to use RegEx in Python. These are some of the essential methods in Python's re module that help with pattern matching, searching, replacing, and extracting data efficiently.
Using re.match() Method
The re.match() method checks if a string matches a given pattern at the beginning. In the example, we will check if the string 'abyss' starts with a pattern that has an 'a' followed by any three characters and ending with an 's'. in case of a match, it will show the message "Search successful."
import re pattern = '^a...s$' test_string = 'abyss' result = re.match(pattern, test_string) if result: print("Search successful.") else: print("Search unsuccessful.")
Output
When you run the program, it will show this output -
Search successful.
Using re.findall() Method
The re.findall() method extracts all occurrences of a pattern in a string. In our example, we will look for all numbers (\d+) in the string 'hello 12 hi 89. Howdy 34' and get them into a list. It then prints all the numbers found.
import re string = 'hello 12 hi 89. Hows you 34' pattern = '\d+' result = re.findall(pattern, string) print("Numbers in the string:", result)
Output
After running the program, you will get this result -
Numbers in the string: ['12', '89', '34']
Using re.search() Method
The re.search() method looks for the first occurrence of a pattern in a string. This example will check if the string "Python is fun" starts with 'Python'. If yes, it displays "Pattern found inside the string."
import re string = "Python is fun" match = re.search('\APython', string) if match: print("Pattern found inside the string.") else: print("Pattern not found.")
Output
This output will be displayed when the program runs -
Pattern found inside the string.
Using re.sub() and re.subn() Methods
The re.sub() method replaces all occurrences of a pattern, while re.subn() does the same but also returns the number of substitutions. In this example, it will remove all spaces (\s+) from the string 'abc 12\ de 23 \n f45 6', which results in 'abc12de23f456'.
import re string = 'abc 12\ de 23 \n f45 6' pattern = '\s+' replace = '' new_string = re.sub(pattern, replace, string) print(new_string)
Output
You will see this result after executing the program -
abc12de23f456
Using re.split() Method
The re.split() method splits a string at matches of the given pattern. Here, it splits the string 'Twelve:12 Eighty nine:89.' at each number (\d+). The output will be a list of parts of the string separated by the numbers.
import re string = 'Twelve:12 Eighty nine:89.' pattern = '\d+' result = re.split(pattern, string) print(result)
Output
The program gives this output when it is run -
['Twelve:', ' Eighty nine:', '.']