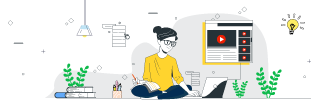
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Catching Base and Derived Classes Exceptions in C++
To catch an exception for both base and derived classes, we need to put the catch block of the derived class before the base class. Otherwise, the catch block for the derived class will never be reached.
This happens because C++ follows a top-down approach when checking catch blocks. So, by placing the derived class catch block first, we ensure that specific exceptions are handled correctly before falling back on the base class.
Algorithm
Following is the algorithm to catch Base and Derived classes Exceptions in C++:
Begin Declare a class B. Declare another class D, which inherits class B. Declare an object of class D. Try: throw derived. Catch (D derived) Print "Caught Derived Exception". Catch (B b) Print "Caught Base Exception". End.
Catching Derived Class Before Base Class
We can handle exceptions in the correct order by placing the catch block for the derived class before the base class.
Example
Here is a simple and correct (order) example where catch of derived class has been placed before the catch of base class, now let us check the output by executing the program:
#include<iostream> using namespace std; class B {}; //class D inherit the class B class D: public B {}; int main() { D derived; try { throw derived; } catch(D derived){ //catch block of derived class cout<<"Caught Derived Exception"; } catch(B b) { //catch block of base class cout<<"Caught Base Exception"; } return 0; }
Following is the output to the above program:
Caught Derived Exception
Catching Base Class Before Derived Class
Here, we are placing the catch block for the base class before the derived class. This is an incorrect practice because base class will catch all matching exceptions first and will not be able to catch derived class expectation.
Example
Here is a simple example where catch of base class has been placed before the catch of derived class:
#include<iostream> using namespace std; class B {}; class D: public B {}; //class D inherit the class B int main() { D derived; try { throw derived; } catch(B b) { cout<<"Caught Base Exception"; //catch block of base class } catch(D derived){ cout<<"Caught Derived Exception"; //catch block of derived class } return 0; }
Following is the output to the above program:
Caught Base Exception
Thus it is proved that we need to put catch block of derived class before the base class. Otherwise, the catch block of derived class will never be reached.