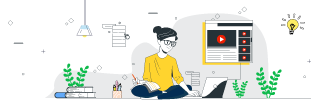
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert List of Objects to JSON Using Gson Library in Java
In this article, we will be discussing how to convert a list of objects to JSON using the Gson library in Java.
JSON is an interchangeable format that is used for data exchange. Values in JSON are represented as key-value pairs. To know more about JSON, refer JSON.
Java GSON Library: Converting a list of objects to JSON
Gson is a third-party Java library developed by Google. It is used for converting Java objects to JSON and vice versa. In the Gson library, we can convert a list of objects to JSON using the toJson() method.
We cannot use the Gson library without downloading or adding it to the project. Because it is not a part of the Java standard library. But we can still use the Gson library without downloading it. We can use it from the Maven repository.
Let's see how to add the Gson library to our project:
- First, we need to add the Gson library to our project. If you are using Maven, add this to your
pom.xml
file:<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.9</version> </dependency>
- If you are not using Maven, add a jar file to your project. You can download the jar file from here.
Steps to convert a list of objects to JSON using the toJson() method:
- First, import the Gson library, as discussed above.
- Then, create a list of objects.
- Then we will create an instance of Gson class.
- Next, we will convert the list to JSON using the toJson() method of Gson.
- Finally, we will print the JSON string.
Example
The Following is the code to convert a list of objects to JSON using the Gson library:
import com.google.gson.Gson; import com.google.gson.GsonBuilder; import java.util.ArrayList; import java.util.List; public class ListToJson { public static void main(String[] args) { // Create a list of objects List<Person> personList = new ArrayList<>(); personList.add(new Person("Ansh", 23)); personList.add(new Person("Bam", 17)); personList.add(new Person("Ace", 22)); // Create a Gson instance Gson gson = new GsonBuilder().setPrettyPrinting().create(); // Convert the list to JSON String json = gson.toJson(personList); // Print the JSON string System.out.println(json); } }
Following is the output of the above code:
[ { "name": "Ansh", "age": 23 }, { "name": "Bam", "age": 17 }, { "name": "Ace", "age": 22 } ]
Using toJsonTree() method
We can also convert a list of objects to JSON using the toJsonTree() method of Gson.
- First, import the Gson library, as discussed above.
- Then, create a list of objects.
- Then we will create an instance of Gson class.
- Next, we will convert the list to JSON using the toJsonTree() method of Gson.
- Then we will convert the JSON array to a string using the toString() method of JsonArray.
- Finally, we will print the JSON string.
Let's see the code to convert a list of objects to JSON using toJsonTree() method:
import com.google.gson.Gson; import com.google.gson.JsonArray; import com.google.gson.JsonElement; import com.google.gson.JsonParser; import java.util.ArrayList; import java.util.List; public class ListToJsonTree { public static void main(String[] args) { // Create a list of objects List<Person> personList = new ArrayList<>(); personList.add(new Person("Ansh", 23)); personList.add(new Person("Bam", 17)); personList.add(new Person("Ace", 22)); // Create a Gson instance Gson gson = new Gson(); // Convert the list to JSON array JsonElement jsonElement = gson.toJsonTree(personList); JsonArray jsonArray = jsonElement.getAsJsonArray(); // Convert the JSON array to string String jsonString = jsonArray.toString(); // Print the JSON string System.out.println(jsonString); } }
Following is the output of the above code:
[ { "name": "Ansh", "age": 23 }, { "name": "Bam", "age": 17 }, { "name": "Ace", "age": 22 } ]