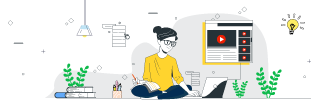
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Calculate Power of a Number in C++
In this article, we'll show you how to calculate the power of a number in C++. Calculating a power means multiplying the base by itself as many times as the exponent indicates. For example, 2 raised to the power of 3 (2^3) means multiplying 2 by itself three times: 2 * 2 * 2, which gives 8.
In C++, there are different ways to calculate the power of a number. Below are the approaches we cover:
Calculate Power of a Number Using Loop
In this approach, we use a while loop to multiply the base number by itself for the exponent number of times. It's a simple and easy-to-understand method.
Example
In this example, we set result to 1 and then multiply it by base in a loop that runs exponent times.
#include <iostream> using namespace std; int power(int base, int exponent) { int result = 1; for (int i = 1; i <= exponent; i++) { result *= base; } return result; } int main() { int base = 2, exponent = 3; // Values directly assigned int result = power(base, exponent); cout << base << " raised to the power " << exponent << " is: " << result << endl; return 0; }
Below is the output for the input values base = 2 and exponent = 3:
2 raised to the power 3 is: 8
Time Complexity: O(n) because we loop n times.
Space Complexity: O(1) because no extra space is used.
Calculate Power of a Number Using Recursion
In this approach, we use recursive to calculate the power of a number. The function calls itself, reducing the exponent each time, until it reaches the base case where the exponent is 0.
Example
In this example, if the exponent is zero, the function returns 1. For any other exponent, the function multiplies the base by the result of the base raised to one less than the current exponent.
#include <iostream> using namespace std; int power(int base, int exponent) { if (exponent == 0) return 1; // Base case return base * power(base, exponent - 1); // Recursive call } int main() { int base = 2, exponent = 3; // Values directly assigned int result = power(base, exponent); cout << base << " raised to the power " << exponent << " is: " << result << endl; return 0; }
The above program outputs the result for base = 2 and exponent = 3.
2 raised to the power 3 is: 8
Time Complexity: O(n) because it makes n recursive calls.
Space Complexity: O(n) because each call adds to the call stack.
Calculate Power of a Number Using pow() Function
In this approach, we make use of the built-in pow() function from the C++ standard library to calculate the power of a number. The pow() function simplifies the process by directly computing the result.
Example
In this example, we simply call the pow() function with the base and exponent as arguments to obtain the result.
#include <iostream> #include <cmath> // For pow() function using namespace std; int main() { int base = 2, exponent = 3; // Values directly assigned int result = pow(base, exponent); cout << base << " raised to the power " << exponent << " is: " << result << endl; return 0; }
The output will be as follows for base = 2 and exponent = 3:
2 raised to the power 3 is: 8
Time Complexity: O(1) because it's a built-in optimized function.
Space Complexity: O(1), no extra space is needed.
Calculate Power of a Number Using Exponentiation by Squaring
This approach makes calculating the power faster by reducing the number of multiplications. It uses the property that:
- base^(2n) = (base^2)^n
- base^(2n+1) = base * (base^2)^n
Example
In this example, we split the exponent and keep squaring the base while halving the exponent each time, which reduces the number of steps needed.
#include <iostream> using namespace std; int power(int base, int exponent) { if (exponent == 0) return 1; // Base case: anything raised to power 0 is 1 if (exponent % 2 == 0) { int halfPower = power(base, exponent / 2); // Recursive call for even exponents return halfPower * halfPower; // Squaring the result } else { return base * power(base, exponent - 1); // Recursive call for odd exponents } } int main() { int base = 2, exponent = 3; int result = power(base, exponent); cout << base << " raised to the power " << exponent << " is: " << result << endl; return 0; }
Below is the output, which shows the result of calculating 2 raised to the power 3 using the given program.
2 raised to the power 3 is: 8
Time Complexity: O(log n) because the exponent is halved each time.
Space Complexity: O(log n) because the call stack grows logarithmically.
Conclusion
In this article, we explained four simple ways to find the power of a number in C++. The loop method is easy but not fast for large numbers. Recursion is cleaner but may fail with large exponents. The pow function is quick and easy to use. Exponentiation by squaring is best for very large exponents because it needs fewer steps.