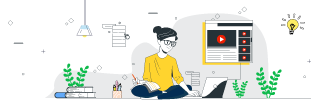
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to Concatenate Two Strings
In this article, we'll show how to write a C++ program to concatenate two strings. A string in C++ is a sequence of characters like letters, numbers, symbols, or anything enclosed in double quotes (e.g., "Hello"). Concatenating two strings means joining them together to form one combined string.
For example, if we have two strings: str1 = "Welcome to" and str2 = "tutorialspoint!", after concatenation, the result will be: result = "Welcome to tutorialspoint!".
Approaches to Concatenate Strings in C++
We can concatenate strings in C++ using different methods. Below are the approaches we will cover:
- Using strcat() Function
- Using a Loop
- Using std::string and the + Operator
- Using std::string::append() Function
Using strcat() Function
In this approach, we use the strcat() function from the <cstring> library to join two null-terminated C-style strings. This function takes the second string and adds it to the end of the first string, modifying the first string directly.
Example
In this example, we define two strings. Then, we use the strcat() function to join the second string to the first one.
#include <iostream> #include <cstring> using namespace std; int main() { char str1[50] = "Welcome to "; char str2[] = "tutorialspoint!"; // Display both the original strings cout << "Original String 1: " << str1 << endl; cout << "Original String 2: " << str2 << endl; // Concatenate str2 to str1 strcat(str1, str2); // Concatenate str2 to str1 // Output the concatenated result cout << "Concatenated String: " << str1 << endl; return 0; }
The output of the above program shows the result of concatenating the two strings.
Original String 1: Welcome to Original String 2: tutorialspoint! Concatenated String: Welcome to tutorialspoint!
Time Complexity: O(n), where n is the length of the first string, as it traverses the first string to find the null terminator.
Space Complexity:O(1), since the operation modifies the string in place without using extra space.
Using a Loop
In this approach, we manually loop through each character of the second string and copy it to the end of the first string. This process continues until we reach the null terminator, after which we add a null terminator to the final string to properly mark its end.
Example
In this example, we find the end of the first string and copy each character from the second string to it. Finally, we add a null terminator to mark the end of the concatenated string.
#include <iostream> using namespace std; int main() { char str1[50] = "Welcome to "; char str2[] = "tutorialspoint!"; int i = 0; // Find the null terminator of str1 while (str1[i] != '\0') { i++; } // concatenating str2 to str1 int j = 0; while (str2[j] != '\0') { str1[i] = str2[j]; i++; j++; } str1[i] = '\0'; // Add null terminator // Display both the original strings cout << "Original String 1: " << "Welcome to " << endl; cout << "Original String 2: " << "tutorialspoint!" << endl; // Output the concatenated result cout << "Concatenated String: " << str1 << endl; return 0; }
Below shows the output of the above program, which shows the concatenation of two strings.
Original String 1: Welcome to Original String 2: tutorialspoint! Concatenated String: Welcome to tutorialspoint!
Time Complexity: O(n + m), where n and m are the lengths of the first and second strings.
Space Complexity: O(n + m), as we need extra space for the concatenated result.
Using std::string and the + Operator
In this approach, we use the + operator with std::string to concatenate two strings. This is a simple and modern method available in C++.
Example
In this example, we define two strings and directly concatenate them into a new string using the + operator.
#include <iostream> #include <string> using namespace std; int main() { string str1 = "Welcome to "; string str2 = "tutorialspoint!"; // Concatenate using + string result = str1 + str2; // Display the original strings and the result cout << "Original String 1: " << str1 << endl; cout << "Original String 2: " << str2 << endl; cout << "Concatenated String: " << result << endl; return 0; }
The output below shows the concatenation of the two strings using the + operator in C++.
Original String 1: Welcome to Original String 2: tutorialspoint! Concatenated String: Welcome to tutorialspoint!
Time Complexity: O(n + m) because it combines two strings of length n and m.
Space Complexity: O(n + m) because a new string of size(n + m) is created to hold the result.
Using std::string::append() Function
In this approach, we use the append() method of std::string to join two strings by adding the second one at the end of the first. This directly modifies the original string.
Example
In this example, we use the append() method to join str2 at the end of str1. Since std::string handles memory internally, we don't need to worry about buffer size or null termination.
#include <iostream> #include <string> using namespace std; int main() { // Original strings related to tutorialspoint string str1 = "Welcome to "; string str2 = "tutorialspoint!"; // Append str2 to str1 using append function str1.append(str2); // Concatenate str2 to str1 // Display the original strings cout << "Original String 1: " << "Welcome to " << endl; cout << "Original String 2: " << "tutorialspoint!" << endl; // Display the concatenated result cout << "Concatenated String: " << str1 << endl; return 0; }
After running the program, you'll see the following output showing the combined strings.
Original String 1: Welcome to Original String 2: tutorialspoint! Concatenated String: Welcome to tutorialspoint!
Time Complexity: O(n + m) because we go through both strings.
Space Complexity: O(1) the operation is done in-place on str1, without creating a new string.
Conclusion
In this article, we covered four methods to concatenate strings in C++. These methods include using the strcat() function, custom loop,the + operator with std::string, and the std::string::append() function. Each method offers a simple way to join strings.