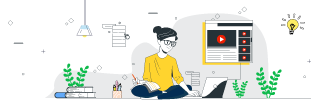
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Display Prime Numbers Between Two Intervals Using Functions in C++
A prime number is a number greater than 1 that has no divisors other than 1 and itself. For example, 2, 3, 5, 7, and 11 are prime numbers. In this article, we'll show you how to write a C++ program to display prime numbers between two intervals using function.
For example, given the interval from 0 to 50, we want to print all the prime numbers within this interval.
Input: Starting interval: 0 Ending interval: 50 Output: Prime numbers in the interval [0, 50] are: 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
Using Functions to Display Prime Numbers Between Two Intervals
To display prime numbers between two intervals, we use a function. A function is a piece of code that performs a specific task and helps keep the code cleaner and more reusable. Here's how we did it:
- First, we defined a function called primeNumbers() that takes two numbers as input, the lower and upper bounds.
- Next, inside this function, we use a loop to go through each number in the interval, skipping 0 and 1 as they are not prime.
- Then, for each number, we check if it is divisible by any number from 2 to half of that number. If not, we print it as a prime number.
- Finally, we call this function from the main() function and pass the interval values.
C++ Program to Display Prime Numbers Between Two Intervals Using Functions
Here's a complete C++ program where we used a function to print all prime numbers between two given intervals.
#include <iostream> using namespace std; // Function to print all prime numbers in the given interval void primeNumbers(int lbound, int ubound) { int flag, i; while (lbound <= ubound) { flag = 0; // 0 and 1 are not prime if (lbound <= 1) { lbound++; continue; } // Check if current number is divisible by any number up to its half for (i = 2; i <= lbound / 2; i++) { if (lbound % i == 0) { flag = 1; // Not a prime break; } } // If no divisor found, it's a prime if (flag == 0) cout << lbound << " "; lbound++; } } int main() { int lowerbound = 0, upperbound = 50; cout << "Starting interval: " << lowerbound << endl; cout << "Ending interval: " << upperbound << endl << endl; cout << "Prime numbers in the interval [" << lowerbound << ", " << upperbound << "] are: " << endl; primeNumbers(lowerbound, upperbound); return 0; }
The output below displays all prime numbers between the given intervals using function.
Starting interval: 0 Ending interval: 50 Prime numbers in the interval [0, 50] are: 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
Time Complexity: O(n^2) because for each number we check up to n/2 divisibility.
Space Complexity: O(1) because no extra space is used apart from a few variables.
Conclusion
In this article, we showed how to write a C++ program using functions to display prime numbers between two intervals. We created a function that checks and displays prime numbers within a specified range, with a time complexity of O(n^2) and space complexity of O(1).