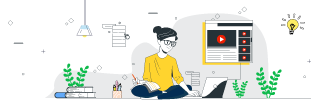
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Kth Smallest Element by Partitioning the Array in C++
In this articles we will find the kth Smallest Element by Partitioning the Array in C++, let's see input/output scenario:
Input / Output Scenario
Following is the input-output scenario:
Input: arr[] = {7, 2, 1, 6, 8, 5, 3, 4} Output: 3
In the above scenario, after sorting the array, it becomes {1, 2, 3, 4, 5, 6, 7, 8}, so the kth smallest element is 3.
An array is a linear data structure that is a collection of elements of the same type in a contiguous memory location. So, to partition a given array, we need to use the quick sort approach.
Quick Sort
Quick sort is a sorting algorithm and is based on the partitioning of an array of data into smaller arrays. It partitions the array into two arrays, one of which holds values smaller than the specified value, say pivot, based on which the partition is made, and another array holds values greater than the pivot value.
Example to Find Kth Smallest Element
The following example finds the Kth Smallest Element by Partitioning the Array in C++:
#include<iostream> using namespace std; void swap(int *a, int *b) { int t; t = *a; *a = *b; *b = t; } int createPartition(int a[], int l, int h) { int pi, in, i; in = l; pi = h; for(i=l; i < h; i++) { if(a[i] < a[pi]) { swap(&a[i], &a[in]); in++; } } swap(&a[pi], &a[in]); return in; } int partition(int a[], int low, int high, int k) { int p_in; if(low < high) { p_in = createPartition(a, low, high); if(p_in == k-1) return k-1; else if(p_in > k-1) return partition(a, low, p_in-1, k); else return partition(a, p_in+1, high, k); } return low; } int main() { int a[] = {7, 2, 1, 6, 8, 5, 3, 4}; int n = sizeof(a) / sizeof(a[0]); int k = 3; int index = partition(a, 0, n-1, k); cout << "\nThe " << k << "rd smallest element: " << a[index] << endl; return 0; }
Following is the kth smallest number is:
The 3rd smallest element: 3