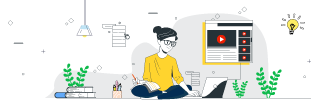
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Median of Elements from Two Different Arrays in C++
The median is defined as the middle value of a sorted list of numbers, and the middle value is found by ordering the numbers in ascending order. Once the numbers are ordered, the middle value is called the median of the given data set.
Here, in this article, we have two different sorted arrays and need to find the median of the array that is formed after merging of two given arrays.
Median depends on the sorted merged array. So, the following cases may occur:
- If the length of the merged array is odd, then the median should be the middle element of the array.
- If the length of the merged array is even, there will be two middle elements. So, the average of both middle elements will be the median of the array.
Input / Output Scenario
The following are the input/output scenario:
Input: arr1[] = [-1, 0, 3, 4, 5], arr2[] = [-2, 5, 7, 8, 9, 10] Output: 5 Explanation: The merged array is [-2, -1, 0, 3 , 4, 5, 5, 7, 8, 9, 10]. So the median of the merged array is 5. Input: arr1[] = [1, 4, 6, 7], arr2[] = [10, 15, 20] Output: 7 Explanation : The merged array is [1, 4, 6, 7, 10, 15, 20]. So the median of the merged array is 7. Input: a[] = [3, 7], b[] = [2, 4, 5, 6] Output: 4.5 Explanation: The merged array is [2, 3, 4, 5, 6, 7]. The total number of elements is even, so there are two middle elements. Average of these two is: (4 + 5) / 2 = 4.5
Find Median Using Naive Approach
The idea behind using the naive approach is to create a new array, concatenate the given two arrays into a new array, sort the new array then return the middle of the new sorted array.
Example
In the following C++ example, we use the naive approach to find the median of the two sorted arrays -
#include <iostream> #include <vector> #include <algorithm> using namespace std; double median_of_2arr(vector < int > & arr1, vector < int > & arr2) { // Merge both the arrays vector < int > arr3(arr1.begin(), arr1.end()); arr3.insert(arr3.end(), arr2.begin(), arr2.end()); // Sort the concatenated array sort(arr3.begin(), arr3.end()); int len = arr3.size(); // If length of array is even if (len % 2 == 0) return (arr3[len / 2] + arr3[len / 2 - 1]) / 2.0; // If length of array is odd else return arr3[len / 2]; } int main() { vector < int > arr1 = {-1, 0, 3, 4, 5}; vector < int > arr2 = {-2, 5, 7, 8, 9, 10}; cout<< "median is: " << median_of_2arr(arr1, arr2) << endl; return 0; }
Following is the median of the above two arrays -
median is: 5
Find Median Using Merge Sort
Both arrays are sorted, so we merge the sorted arrays efficiently and count the total number of elements in the merged array. So, when we achieved half of the total, print the median. There can be two cases:
- Case 1: If the m+n is odd, the median is the ((m+n)/2)th element while merging the array.
- Case 2: If the m+n is even, the median is the average of the ((m+n)/2 -1)th and ((m+n)/2)th elements while merging the array.
Example
In the following C++ example, we use the merger sort to find the median of the two sorted arrays -
#include <iostream> #include <vector> using namespace std; double median_of_2arr(vector < int > & arr1, vector < int > & arr2) { int n = arr1.size(), m = arr2.size(); int i = 0, j = 0; // m1 and m2 to store middle and second middle element int m1 = -1, m2 = -1; for (int count = 0; count <= (m + n) / 2; count++) { m2 = m1; // If both the arrays have remaining elements if (i != n && j != m) m1 = (arr1[i] > arr2[j]) ? arr2[j++] : arr1[i++]; // If only arr1[] has remaining elements else if (i < n) m1 = arr1[i++]; // If only arr2[] has remaining elements else m1 = arr2[j++]; } if ((m + n) % 2 == 1) return m1; else return (m1 + m2) / 2.0; } int main() { vector < int > arr1 = {3, 7}; vector < int > arr2 = {2, 4, 5, 6}; cout<< "median is: " << median_of_2arr(arr1, arr2) << endl; return 0; }
Following is the median of the above two arrays -
median is: 4.5