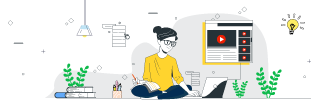
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Implement Double Order Traversal of a Binary Tree in C++
Double Order Traversal
Double order traversal means each node in a tree is traversed twice in a particular order.
A binary tree is a non-linear data structure where each node contains at most two children (i.e, left and right). Therefore, suppose we have a given binary tree, and the task is to find its double-order traversal. A double order traversal is a tree traversal technique in which every node traverses twice in the following order:
- Visit the node.
- Traverse the left subtree.
- Visit the node.
- Traverse the right subtree.
Let's see a Diagram of the binary tree, then we traverse in a double order:

Following is the double order traversal of the above binary tree: 23553266
Steps to Implement Double Order Traversal in Binary Tree
Following are the steps:
- Start the inorder traversal from the root.
- If the current node does not exist simply return from it.
- Otherwise, store the value of the current node. Recursively traverse the left subtree. Again, store the current node. Recursively traverse the right subtree.
- Repeat the above steps until all nodes in the tree are visited.
C++ Program to Implement Double Order Traversal of a Binary Tree
In the following example, we implement the double order traversal of a binary tree:
#include <bits/stdc++.h> using namespace std; class Node { public: int data; Node * left; Node * right; Node(int x) { data = x; left = nullptr; right = nullptr; } }; // Double order traversal vector < int > double_order_traversal(Node * root) { vector < int > result; if (!root) return result; // Store node value before traversing result.push_back(root -> data); // Recursively traverse the left subtree vector < int > leftSubtree = double_order_traversal(root -> left); result.insert(result.end(), leftSubtree.begin(), leftSubtree.end()); // Store node value again after // traversing left subtree result.push_back(root -> data); // Recursively traverse the right subtree vector < int > rightSubtree = double_order_traversal(root -> right); result.insert(result.end(), rightSubtree.begin(), rightSubtree.end()); return result; } int main() { Node * root = new Node(2); root -> left = new Node(3); root -> right = new Node(6); root -> left -> left = new Node(5); vector < int > result = double_order_traversal(root); for (int res: result) { cout << res << " "; } return 0; }
Following is the output of the above code:
2 3 5 5 3 2 6 6
Advertisements