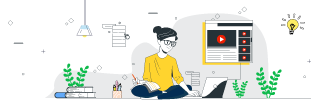
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Implement Sorted Circularly Doubly Linked List in C++
Sorted Circular Doubly Linked List
A sorted circular doubly linked list is a type of circular doubly linked list in which elements are arranged in a specific order, typically ascending or descending based on the data values. The insertion operation makes sure that the new node is placed in its correct sorted position.
In Circular Doubly Linked List two consecutive elements are linked or connected by previous and next pointer and the last node points to first node by next pointer and the first node also points to last node by previous pointer.

Characteristics of Sorted Circular Doubly Linked List
The following are the characteristics of the circular doubly linked list:
- Sorted: All the inserted node will be in sorted order either in ascending or descending order.
- Circular: The main feature is that it is circular in design.
- Doubly Linked: Each node in a circular linked list has two pointers. (i.e., next and previous).
- Header Node: A circular doubly linked list has a header node, which is frequently used to make execution of a certain operation.
Example of Sorted Circular Double Linked List
Following is the C++ implementation of the Sorted circular doubly linked list to insert the element and display them:
#include<iostream> #include<cstdio> #include<cstdlib> using namespace std; struct nod { int info; struct nod * n; struct nod * p; }* start, * last; int count = 0; class circulardoublylist { public: nod * create_node(int); void insert_sorted(int); void display(); circulardoublylist() { start = NULL; last = NULL; } }; // Function to create a new node nod * circulardoublylist::create_node(int v) { count++; nod * t = new nod; t -> info = v; t -> n = t -> p = NULL; return t; } // Function to insert a node while maintaining sorted order void circulardoublylist::insert_sorted(int v) { nod * t = create_node(v); // If the list is empty if (start == NULL) { start = last = t; start -> n = start; start -> p = start; return; } // If the new node should be the new head if (v < start -> info) { t -> n = start; t -> p = last; last -> n = t; start -> p = t; start = t; return; } // Insert at correct position nod * s = start; while (s -> n != start && s -> n -> info < v) { s = s -> n; } t -> n = s -> n; t -> p = s; s -> n -> p = t; s -> n = t; if (s == last) { last = t; } } // Function to display the list void circulardoublylist::display() { if (start == NULL) { cout << "The List is empty, nothing to display" << endl; return; } nod * s = start; do { cout << s -> info << " <-> "; s = s -> n; } while (s != start); cout << endl; } int main() { circulardoublylist cdl; int predefined_values[] = {5, 10, 3, 8, 12, 7}; for (int v: predefined_values) { cdl.insert_sorted(v); } cout << "Sorted Circular Doubly Linked List:" << endl; cdl.display(); return 0; }
Following is the output:
Sorted Circular Doubly Linked List: 3 <-> 5 <-> 7 <-> 8 <-> 10 <-> 12 <->
Advertisements