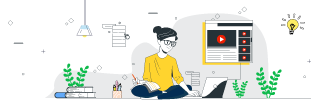
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to Implement Ternary Tree
A ternary tree is a type of tree data structure in which each node can have at most three children. In this article, we will learn how to implement a ternary tree in C++ using basic class structures and pointers.
What is Ternary Tree?
A ternary tree is a tree in which each node has up to three children, that is left, middle, and right. It is same as a binary tree, but with an extra child node for each node. Each node in a ternary tree stores a data value and pointers to its three child nodes.
For example, a node in a ternary tree will be defined by:
struct Node { int data; Node* left; Node* middle; Node* right; };
Implementing Ternary Tree
To implement a ternary tree, we define a class or structure for nodes and use recursive logic for inserting and traversing. Below are some points about its structure and operation:
- Structure: Each node contains a data value and three pointers for left, middle, and right child.
- Operations: Insert nodes and perform traversal operation (preorder, inorder, or postorder).
Steps to Implement Ternary Tree in C++
Following are steps/algorithm to implement ternary tree in C++:
- Create a structure or class for tree nodes.
- Each node should have three pointers: left, middle, and right.
- Insert values into the tree using arrow operator (->) for pointers.
- Use a preorder traversal to display values of tree.
- Use a main function to create nodes and demonstrate traversal.
C++ Program to Implement Ternary Tree
In the below code, we have implemented a simple ternary tree and traversed it using preorder traversal:
#include <iostream> using namespace std; // Define a node of the ternary tree struct Node { int data; Node* left; Node* middle; Node* right; Node(int val) { data = val; left = middle = right = nullptr; } }; // Preorder traversal of ternary tree void preorder(Node* root) { if (root == nullptr) return; cout << root->data << " "; preorder(root->left); preorder(root->middle); preorder(root->right); } int main() { // Creating root and child nodes Node* root = new Node(1); root->left = new Node(2); root->middle = new Node(3); root->right = new Node(4); root->left->left = new Node(5); root->middle->middle = new Node(6); root->right->right = new Node(7); cout << "Preorder Traversal: "; preorder(root); return 0; }
The output of above code will be:
Preorder Traversal: 1 2 5 3 6 4 7
Time and Space Complexity
Time Complexity: O(n), where n is the number of nodes in the ternary tree.
Space Complexity: O(h), where h is the height of the tree due to recursion stack.