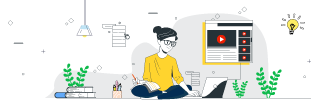
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to Implement String Search Algorithm for Short Text Sizes
Searching for a substring within a short text is a common operation in many C++ programs, like as small-scale text editors, command line utilities, and education projects. So, in this article, we will implement a string search algorithm for short text sizes.
Importance of String Search
String search algorithms find a substring within another string. While advanced methods like KMP and Boyer-Moore are great for longer texts, a simple method like Naive search works well for short texts without added complexity.
Algorithm to Implement String Search Algorithm for Short Text Sizes
The Following is a string search algorithm ?
Begin Take the string and pattern as input. Declare the original and duplicate array with their size. Put the lengths of original and duplicate in len_ori and len_dupli. Make a loop for find out the position of the searched pattern. If pattern is not found, print not found otherwise print the no of instances of the searched pattern. End
C++ Program to Implement String Search Algorithm
The following examle, searches for all occurrences of a predefined substring (pattern) within a given original string using a basic character-by-character comparison (naive string search).
#include<iostream> #include<cstring> using namespace std; int main() { char ori[120] = "hellotutorialspoint"; char dupli[120] = "hello"; int i, j, k = 0; int len_ori = strlen(ori); int len_dupli = strlen(dupli); cout << "Original String: " << ori << endl; cout << "Pattern to Search: " << dupli << endl; for (i = 0; i <= (len_ori - len_dupli); i++) { for (j = 0; j < len_dupli; j++) { if (ori[i + j] != dupli[j]) { break; } } if (j == len_dupli) { k++; cout << "\nPattern Found at Position: " << i; } } if (k == 0) { cout << "\nNo Match Found!"; } else { cout << "\nTotal Instances Found = " << k << endl; } return 0; }
Output
When the above code is compiled and executed, it produces the following result ?
Original String: hellotutorialspoint Pattern to Search: hello Pattern Found at Position: 0 Total Instances Found = 1