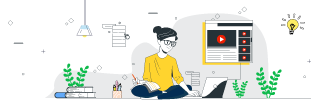
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to Implement Vector in STL
Vector is a special type of array in C++, which have ability to resize itself automatically during insertion and deletion of elements.. In this article, we will learn how to use the vector container from the Standard Template Library (STL) in C++.
What is a Vector?
A vector is a container that stores data elements in a dynamic contiguous memory. It is similar to arrays but can change its size dynamically during execution. Meaning, we doesn't need explicitly mention the size of a vector, it will be calculated automatically by counting number of elements in it. Similar to arrays, vectors are also indexed starting from 0. The STL library of C++ provides a pre-defined vector container that allows random access and efficient insertions and deletions at the end.
For example, in the code we have shown how data are inserted and accessed in vector:
// Declare a vector vector<int> v; // Add Data v.push_back(10); v.push_back(20); cout << v[0]; // Will print 10
Using vector Container in STL
The vector container is defined in the <vector> header of STL. It provides a dynamic array-like structure with various member functions. Below are some points about this container:
- Header: <vector>
-
Syntax:
vector<datatype> vector_name;
- Common functions:
- push_back() - Function used to insert an element at the end of the vector.
- pop_back() - Function used to remove the last element of the vector.
- size() - Function used to return the number of elements in the vector.
- empty() - Function used to check if the vector is empty.
- at() - Function used to access element at specific position with bounds checking.
- front() - Function used to access the first element of the vector.
- back() - Function used to access the last element of the vector.
Steps to Implement Vector in C++ STL
Following are steps/algorithm to use vector using C++ STL:
- Include the <vector> header file.
- Declare a vector with desired data type.
- Use push_back() to insert elements at the end.
- Access elements using [] or at().
- Use pop_back() to remove the last element.
- Use front() and back() to access boundary elements.
- Use size() and empty() to check status.
C++ Program to Implement Vector using STL
The below code is the implementation of the above algorithm in C++ language.
#include <iostream> #include <vector> using namespace std; int main() { vector<int> v; // Insert elements v.push_back(10); v.push_back(20); v.push_back(30); cout << "First Element: " << v.front() << endl; cout << "Last Element: " << v.back() << endl; // Access elements using index cout << "Element at index 1: " << v[1] << endl; // Remove last element v.pop_back(); cout << "After pop_back operation:" << endl; cout << "Vector Size: " << v.size() << endl; // Check if empty if (v.empty()) { cout << "Vector is empty" << endl; } else { cout << "Vector is not empty" << endl; } return 0; }
The output of above code will be:
First Element: 10 Last Element: 30 Element at index 1: 20 After pop_back operation: Vector Size: 2 Vector is not empty
Time and Space Complexity
- push_back(), pop_back(), front(), back(): O(1) for each operation on average.
- at(), []: O(1) for direct access.
Space Complexity: O(n), where n is the number of elements in the vector.