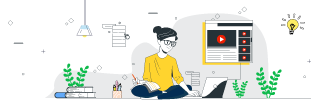
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sort Elements in Lexicographical Order in C++
Lexicographical order denotes the way the words are ordered in a list, based on alphabetical order according to their alphabets. For example:
List of words: Harry Adam Sam Lexicographical order of words: Adam Harry Sam
Sorting String Based on Lexicographical Order
To implement the string in lexicographical order, use the two different iterators: one to point at the current string, and the other to compare it with the next strings in the array. If the first iterator points to a string that is greater than the one pointed to by the second iterator, then we swap the two strings. This process continues until all the strings are sorted in lexicographical (dictionary) order. After the sorting is done, we simply use a loop or iterator to print the strings one by one in the sorted order.
C++ Program to Sort Elements in Lexicographical Order
This program demonstrates the sorting of strings in a lexicographical sort:
#include <iostream> using namespace std; int main() { int i, j; string s[5] = {"Harry", "Adam", "Sam", "Alia", "Zara"}; string temp; // Lexicographical sorting for(i = 0; i < 4; ++i) { for(j = i + 1; j < 5; ++j) { if(s[i] > s[j]) { temp = s[i]; s[i] = s[j]; s[j] = temp; } } } cout << "The elements in lexicographical order are" << endl; for(i = 0; i < 5; ++i) cout << s[i] << endl; return 0; }
The above program produces the following output:
The elements in lexicographical order are Adam Alia Harry Sam Zara