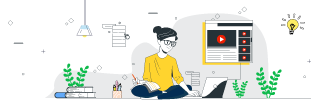
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create New Instance of a Two-Dimensional Array with Java Reflection Method
A new instance of a two dimensional array can be created using the java.lang.reflect.Array.newInstance() method. This method basically creates the two-dimensional array with the required component type as well as length.
A program that demonstrates the creation of a two-dimensional array using the Array.newInstance() method is given as follows −
Example
import java.lang.reflect.Array; public class Demo { public static void main (String args[]) { int size[] = {3, 3}; int arr[][] = (int[][])Array.newInstance(int.class, size); System.out.println("The two-dimensional array is:"); for(int[] i: arr) { for(int j: i ) { System.out.print(j + " "); } System.out.println(); } } }
Output
The two-dimensional array is: 0 0 0 0 0 0 0 0 0
Now let us understand the above program. A new instance of a two-dimensional array is created using the Array.newInstance() method. A code snippet which demonstrates this is as follows −
int size[] = {3, 3}; int arr[][] = (int[][])Array.newInstance(int.class, size);
Then the array elements are displayed using the nested for loop. A code snippet which demonstrates this is as follows −
System.out.println("The two-dimensional array is:"); for(int[] i: arr) { for(int j: i ) { System.out.print(j + " "); } System.out.println(); }
Advertisements