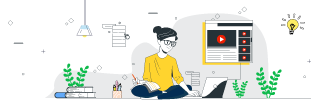
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Declarations in Python Class vs. init Method
In Python, the declarations within a class are not equivalent to those within the __init__() method.
Let's discuss the class and __init__() method. A class is a collection of objects. It contains the blueprints or the prototype from which the objects are being created. It is a logical entity that contains some attributes and methods.
Python '__init__()'
The Python __init__() function is one of the OOP's concepts. The __init__() is called automatically whenever the object is been created to a class.
The __init__() function is also known as a constructor. Constructors are used to initialize the objects. The task of constructors is to initialize (assign values to) the data members of the class when an object of the class is created.
Declaration of class
A class is created by using the keyword class, and it is followed by a class name, which should follow Pascal case [each word's first letter should be capitalized]. Following is the syntax of the declaration of a class -
class ClassName: statements
Example
In the following example, we have declared a class of Animal and called by an object obj1 -
class Animal: print("I am Animal") obj1=Animal()
Following is the output of the above code ?
I am Animal
Declaration of __init__()
Following is the syntax of __init__() method in Python -
class ClassName: def __init__(self): statement1 statement2
Example
With the following example, let's understand the implementation of the __init__() method. Here, when we created an object the method automatically called -
class Tutorialspoint: def __init__(self): print("Hello welcome to Python course") obj1=Tutorialspoint()
Following is the output of the above code -
Hello welcome to Python course
Class Attributes Vs Instance Attributes
From the above discussion, we have got a basic idea of class and the __init__() method. Now let's declare the attributes inside the class. Attributes are nothing but variables declared inside the class. There are two types of attributes based on the declaration -
- Class Attributes: The attributes that are defined globally in the class are known as class attributes and can be accessed throughout the class itself and are shared between all class instances. They can be accessed using the class name.
- Instance Attributes: The attributes that are defined inside the __init__() method are known as instance attributes. These are specific to the current instance (object) and are not shared with other class instances. We can access the instance attributes using object name.
Example
In the following example, we can find the difference between class attributes and instance attributes -
class Student: # Class Attribute school_name = "Oxword" def __init__(self, name, grade): # Instance Attributes self.name = name self.grade = grade # Creating two student objects s1 = Student("John", "8th") s2 = Student("Annie", "9th") # Accessing attributes print(s1.name, s1.grade, s1.school_name) print(s2.name, s2.grade, s2.school_name) # Changing class attribute using class name Student.school_name = "Maple Leaf School" # Changing instance attribute s1.name = "Roshan" # Displaying updated values print(s1.name, s1.grade, s1.school_name) print(s2.name, s2.grade, s2.school_name)
Following is the output of the above code -
John 8th Oxword Annie 9th Oxword Roshan 8th Maple Leaf School Annie 9th Maple Leaf School