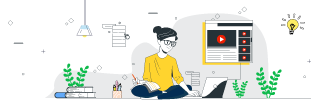
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create a Class for Basic Calculator Operations in Golang
To create a class that can perform basic calculator operations, we can take following Steps
- We can define a Calculator class with two numbers, a and b.
- Define a member method to calculate the addition of two numbers.
- Define a member method to calculate the multiplication of two numbers.
- Define a member method to calculate the division of two numbers.
- Define a member method to calculate the subtraction of two numbers.
- In the main method, declare two variables, a and b.
- Get an instance of Calculator.
- Initialize a choice variable, based on which mathematical operations could be performed.
Example
package main import ( "fmt" ) type Calculator struct { a int b int } func (c *Calculator)Add(){ fmt.Println("Addition of two numbers: ", c.a + c.b) } func (c *Calculator)Mul(){ fmt.Println("Multiplication of two numbers: ", c.a * c.b) } func (c *Calculator)Div(){ fmt.Println("Division of two numbers: ", c.a / c.b) } func (c *Calculator)Sub(){ fmt.Println("Subtraction of two numbers: ", c.a - c.b) } func main(){ var a, b int fmt.Print("Enter the first number: ") fmt.Scanf("%d", &a) fmt.Print("Enter the second number: ") fmt.Scanf("%d", &b) cal := Calculator{ a: a, b: b, } c:=1 for c>=1 { fmt.Println("Enter 1 for Addition: ") fmt.Println("Enter 2 for Multiplication: ") fmt.Println("Enter 3 for Division: ") fmt.Println("Enter 4 for Subtraction: ") fmt.Print("Enter 5 for Exit: ") fmt.Scanf("%d", &c) switch c { case 1: cal.Add() case 2: cal.Mul() case 3: cal.Div() case 4: cal.Sub() case 5: c = 0 break default: fmt.Println("Enter valid number.") } } }
Output
Enter the first number: 7 Enter the second number: 3 Enter 1 for Addition: Enter 2 for Multiplication: Enter 3 for Division: Enter 4 for Subtraction: Enter 5 for Exit: 1 Addition of two numbers: 10 Enter 1 for Addition: Enter 2 for Multiplication: Enter 3 for Division: Enter 4 for Subtraction: Enter 5 for Exit: 2 Multiplication of two numbers: 21 Enter 1 for Addition: Enter 2 for Multiplication: Enter 3 for Division: Enter 4 for Subtraction: Enter 5 for Exit: 3 Division of two numbers: 2 Enter 1 for Addition: Enter 2 for Multiplication: Enter 3 for Division: Enter 4 for Subtraction: Enter 5 for Exit: 4 Subtraction of two numbers: 4 Enter 1 for Addition: Enter 2 for Multiplication: Enter 3 for Division: Enter 4 for Subtraction: Enter 5 for Exit: 5
Advertisements