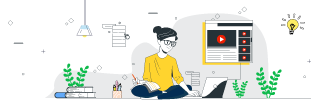
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Merge Two JSON Arrays in Java
In this article, we will be learning how to merge two JSON arrays in Java. Let's understand step by step. First, if you are not familiar with JSON, refer JSON overview.
How to Merge Two JSON Arrays in Java?
There are multiple ways to merge two JSON arrays in Java. Some of them are:
- Using org.json library: We will use JSONArray.put() method to merge two JSON arrays.
- Using Gson library: We will use JsonArray.add() method to merge two JSON arrays.
- Using Jackson library: We will use ObjectMapper.createArrayNode() method to merge two JSON arrays.
Let's look at all of them in detail.
Using the org.json library
Let's see how to merge two JSON arrays using the org.json library. The org.json is a library that works with JSON data in Java. It provides classes and methods to create, parse, and manipulate JSON data.
Follow the steps below:
- First, we will need to add the org.json library. We can either download it from its official website or include it in our project if we are using Maven or Gradle. If you are using Maven, add this to your
pom.xml
file:<dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20210307</version> </dependency>
- Next, we will create two JSON arrays.
- Then, we will merge the two JSON arrays using the
JSONArray.put()
method. - Finally, we will print the merged JSON array.
Example
Below is the code demonstrating how to merge two JSON arrays using the org.json library:
import org.json.JSONArray; import org.json.JSONObject; public class MergeJsonArraysEx{ public static void main(String[] args){ // Create two JSON arrays JSONArray jsonArray1 = new JSONArray(); jsonArray1.put(new JSONObject().put("name", "Ansh").put("age", 22)); jsonArray1.put(new JSONObject().put("name", "John").put("age", 25)); jsonArray1.put(new JSONObject().put("name", "Vishwa").put("age", 12)); JSONArray jsonArray2 = new JSONArray(); jsonArray2.put(new JSONObject().put("name", "Amit").put("age", 30)); jsonArray2.put(new JSONObject().put("name", "Ravi").put("age", 28)); jsonArray2.put(new JSONObject().put("name", "Sita").put("age", 20)); // Merge the two JSON arrays JSONArray mergedArray = new JSONArray(); for(int i = 0; i < jsonArray1.length(); i++){ mergedArray.put(jsonArray1.get(i)); } for(int i = 0; i < jsonArray2.length(); i++){ mergedArray.put(jsonArray2.get(i)); } // Print the merged JSON array System.out.println("Merged JSON Array: " + mergedArray.toString(2)); } }
Following is the output of the above code:
Merged JSON Array: [ { "name": "Ansh", "age": 22 }, { "name": "John", "age": 25 }, { "name": "Vishwa", "age": 12 }, { "name": "Amit", "age": 30 }, { "name": "Ravi", "age": 28 }, { "name": "Sita", "age": 20 } ]
Using Gson library
Now, let's see how to merge two JSON arrays using the Gson library. Gson is a popular library developed by Google for converting Java objects to JSON and vice versa.
To use Gson in your code, you will need to add it to your project. You can simply download it from the official website or if you are using Maven, add this to your pom.xml
file:
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.9</version> </dependency>
Steps to follow to merge two JSON arrays using Gson:
- As discussed above, add the Gson library.
- Create two JSON arrays.
- Merge the two JSON arrays using the
JsonArray.add()
method. - Print the merged JSON array.
Example
Now, let's see how to merge two JSON arrays using the Gson library:
import com.google.gson.Gson; import com.google.gson.JsonArray; import com.google.gson.JsonObject; import com.google.gson.JsonParser; import com.google.gson.JsonElement; import java.util.ArrayList; import java.util.List; import java.util.Iterator; import java.util.Map; public class MergeJsonArraysGsonEx{ public static void main(String[] args){ // Create two JSON arrays String jsonArray1 = "[{"name":"Ansh","age":22},{"name":"John","age":25},{"name":"Vishwa","age":12}]"; String jsonArray2 = "[{"name":"Amit","age":30},{"name":"Ravi","age":28},{"name":"Sita","age":20}]"; // Parse the JSON arrays JsonParser parser = new JsonParser(); JsonArray array1 = parser.parse(jsonArray1).getAsJsonArray(); JsonArray array2 = parser.parse(jsonArray2).getAsJsonArray(); // Merge the two JSON arrays JsonArray mergedArray = new JsonArray(); for(JsonElement element : array1){ mergedArray.add(element); } for(JsonElement element : array2){ mergedArray.add(element); } // Print the merged JSON array System.out.println("Merged JSON Array: " + mergedArray.toString()); } }
Output
Following is the output of the above code:
Merged JSON Array: [{"name":"Ansh","age":22},{"name":"John","age":25},{"name":"Vishwa","age":12},{"name":"Amit","age":30},{"name":"Ravi","age":28},{"name":"Sita","age":20}]
Using Jackson library
Now, let's see how to merge two JSON arrays using the Jackson library. Jackson is a popular library for processing JSON data in Java. It is simpler and faster than other libraries.
To use Jackson in your code, you will need to add it to your project. You can simply download it from the official website or if you are using Maven, add this to your pom.xml
file:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.12.3</version> </dependency>
Steps to follow to merge two JSON arrays using Jackson:
- As discussed above, add the Jackson library.
- Create two JSON arrays.
- Merge the two JSON arrays using the
ObjectMapper
class. - Print the merged JSON array.
Example
Below is the code demonstrating how to merge two JSON arrays using the Jackson library:
import com.fasterxml.jackson.databind.JsonNode; import com.fasterxml.jackson.databind.ObjectMapper; public class MergeJsonArraysJacksonEx{ public static void main(String[] args) throws IOException{ // Create two JSON arrays String jsonArray1 = "[{"name":"Ansh","age":22},{"name":"John","age":25},{"name":"Vishwa","age":12}]"; String jsonArray2 = "[{"name":"Amit","age":30},{"name":"Ravi","age":28},{"name":"Sita","age":20}]"; // Create ObjectMapper instance ObjectMapper objectMapper = new ObjectMapper(); // Parse the JSON arrays JsonNode array1 = objectMapper.readTree(jsonArray1); JsonNode array2 = objectMapper.readTree(jsonArray2); // Merge the two JSON arrays ArrayNode mergedArray = objectMapper.createArrayNode(); for(JsonNode element : array1){ mergedArray.add(element); } for(JsonNode element : array2){ mergedArray.add(element); } // Print the merged JSON array System.out.println("Merged JSON Array: " + mergedArray.toString()); } }
Output
Following is the output of the above code:
Merged JSON Array: [{"name":"Ansh","age":22},{"name":"John","age":25},{"name":"Vishwa","age":12},{"name":"Amit","age":30},{"name":"Ravi","age":28},{"name":"Sita","age":20}]