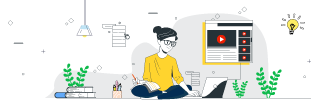
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert Between List and Array in Java
In Java, a List is an interface that represents an ordered collection of elements of the same type. You cannot directly create an object of the List interface, instead, you need to instantiate an ArrayList class or another class that implements the List interface.
An array is a container that holds a fixed size of similar types of data. As the size is fixed, it can not be changed once created.
The conversion between List and array is important because array provides faster access to the elements than List interface (faster in case of searching elements), and provides a fixed size of the elements, which cannot be changed (good for security purposes), so in such cases, using array is a better option.
Converting a List to an Array using toArray() Method
Using the toArray() method, we can convert a list to an Array. This method returns an array containing all of the elements in the current list in a proper sequence.
Following is the syntax of the toArray() method:
ArrayList.toArray(Integer[] a)
Here, a is an array into which the elements of the list are to be stored.
Example
In the following example, we use the toArray() method to convert and retrieve an array that contains all of the list elements {10, 20, 30}:
import java.util.ArrayList; public class ListToArray { public static void main(String args[]) { //creating a list ArrayList<Integer> list = new ArrayList<>(); list.add(10); list.add(20); list.add(30); System.out.println("The list elements are: " + list); //create an array Integer arr[] = new Integer[list.size()]; //using toArray() method list.toArray(arr); // fill the array System.out.print("An array after converting: " ); for(int i = 0; i<arr.length; i++){ System.out.print(arr[i] + " "); } } }
The above program produces the following output:
The list elements are: [10, 20, 30] An array after converting: 10 20 30
Using Iteration
Using iteration, we can not directly convert a list to an Array, but we can iterate through the list elements, and using the assignment '=' operator and the get() method, we assign all the list elements to the array one by one.
That array will be considered as the converted array, which has the same elements as the list.
Following is the syntax of the get() method:
ArrayList.get(int index);
Here, the index is the position of an element in the list.
The Assignment '=' operator assigns values from the right side operands to the left side operand.
Example
In the example below, we use the assignment '=' operator and get() method inside the for loop (for iteration) to assign each list element to an array so that the array has the same elements as the list:
import java.util.ArrayList; public class ListToArray { public static void main(String args[]) { //creating a list ArrayList<String> fruit_list = new ArrayList<>(); fruit_list.add("Apple"); fruit_list.add("Banana"); fruit_list.add("Orange"); fruit_list.add("Grapes"); System.out.println("The list elements are: " + fruit_list); //create an array String fruit_arr[] = new String[fruit_list.size()]; //iterating through list for(int i = 0; i<fruit_list.size(); i++){ //using assignment operator and get() method fruit_arr[i] = fruit_list.get(i); } System.out.print("Array after converting: "); for(int i = 0; i<fruit_arr.length; i++){ System.out.print(fruit_arr[i] + " "); } } }
Following is the output of the above program:
The list elements are: [Apple, Banana, Orange, Grapes] Array after converting: Apple Banana Orange Grapes