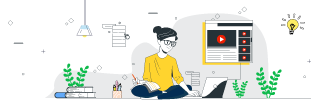
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create a Thread Using Anonymous Class in Java
This article will use an anonymous class to create a Thread in Java. An Anonymous class is a class that does not have a name.
Thread in Java
In Java, a Thread is a part of a program that can be executed independently. All Java programs have at least one thread, known as the main thread, which is created by the Java Virtual Machine (JVM), when the main() method is invoked.
Creating a Thread by Using Anonymous Class
In Java, the basic way to create a thread is to either extend the Thread class or implement the Runnable interface. However, we can also create a thread using an anonymous inner class without extending the Thread class.
Syntax of Creating an Anonymous Class in Java:
Following is the syntax to create an Anonymous Class in Java:
new ClassOrInterfaceName() { // Class body (methods or fields) };
Here, the new keyword is used to create an instance of the anonymous class that either extends a Thread class or implements the Runnable interface.
Example
In the following example, we use the anonymous class new Thread() to create a thread by extending the Thread class anonymously and overriding its run() method:
public class AnonymousThreadTest { public static void main(String[] args) { //anonymous class new Thread() { public void run() { for (int i=1; i <= 5; i++) { System.out.println("Anonymous Thread run() method: " + i); } } }.start(); for (int j=1; j <= 5; j++) { System.out.println("main() method: " + j); } } }
The above program produces the following output:
main() method: 1 main() method: 2 main() method: 3 Anonymous Thread run() method: 1 main() method: 4 Anonymous Thread run() method: 2 main() method: 5 Anonymous Thread run() method: 3 Anonymous Thread run() method: 4 Anonymous Thread run() method: 5
Note! The output may vary each time you compile and run the program.