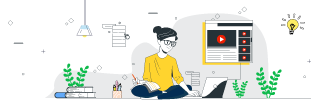
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Handle ArrayStoreException Unchecked in Java
In Java, ArrayStoreException is a public class that extends the RuntimeException class of the java.lang package. It is thrown by the Java Virtual Machine when a runtime error occurs. Since it is an unchecked exception, it does not require an explicit declaration in a method or a constructor's throws clause.
Let's understand why the ArrayStoreException is thrown and how we can avoid it.
Reason for ArrayStoreException in Java
As mentioned earlier, ArrayStoreException is an unchecked exception, and it can occur when we try to store an object of one type in an array of a different type. Usually, one would come across this error when an integer is stored in an array of a different type, such as an array of String or Float.
Example: Throwing ArrayStoreException
In the below Java program, we are trying to store an Integer object in an array of Float type.
public class ArrayStoreExceptionTest { public static void main(String[] args) { Object[] names = new Float[2]; names[1] = new Integer(2); } }
Output
On executing the above code, it will throw java.lang.ArrayStoreException as shown below:
Exception in thread "main" java.lang.ArrayStoreException: java.lang.Integer at ArrayStoreExceptionTest.main(ArrayStoreExceptionTest.java:4)
How to handle ArrayStoreException?
We can handle the ArrayStoreException using try and catch blocks. In addition to this, it is better to follow some practices to avoid such exception or runtime error. A few of them are as follows:
- Write the code that can throw ArrayStoreException within try block and handle it in corresponding catch block.
- Be more specific with the type of array. Avoid declaring the array of Object type, instead use specific class, such as String or Integer.
- Use isInstance() method to check if the type of array is the same as declared before assigning value.
Example: Handling ArrayStoreException
Here, we will use the isInstance in if conditional statement with try-catch block to avoid the ArrayStoreException:
public class ArrayStoreExceptionTest { public static void main(String[] args) { // Array declaration Object[] names = new Float[2]; try { Integer value = Integer.valueOf(2); // Checking array type and value if (Float.class.isInstance(value)) { names[1] = value; } else { System.out.println("Incompatible type"); } } catch (ArrayStoreException e) { e.printStackTrace(); System.out.println("ArrayStoreException is handled"); } System.out.println("Continuing with the statements after try and catch blocks"); } }
Output
The above Java code prints the message to indicate incompatible type value is being assigned:
Incompatible type Continuing with the statements after try and catch blocks