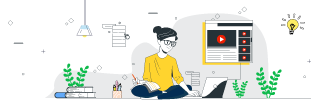
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Read External JSON File in JavaScript
There are three effective methods to read JSON files in JavaScript using fetch(), import statements, and require(). Please check this article which includes examples and outputs with the approach explanation.
JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy for humans to read and write and easy for machines to parse and generate. In this article, we'll explore three different approaches to reading JSON files in JavaScript:
Approaches to Read JSON file
Each method has its use cases and considerations, so let's dive into each approach.
JSON File
This will be our JSON file(data.json). We are going to use this same file in all three approaches.
{ "users":[ { "site":"Tutorialspoint", "user": "Simple and Easy Learning" } ] }
Using the fetch() Method
The fetch() method is a modern way to make network requests in JavaScript. It's widely supported in browsers and can be used in Node.js with a polyfill.
The fetch() method returns a Promise that resolves with the Response object representing the response to the request. We can then use the json() method to parse the response body as JSON.
Example
// File name data.json in the same directory fetch('./data.json') .then(response => response.json()) .then(data => { console.log(data); }) .catch(error => { console.error('Error:', error); });
Output
If the JSON file is not on the server then it will throw an error as shown below. So we recommend you to use this when your JSON file is on the server.
Using the import Statement
The import statement is part of ECMAScript 6 (ES6) and allows you to import JSON files directly as modules. This approach is supported in modern browsers and in Node.js (version 13.2.0 and later with the --experimental-json-modules flag).
When using the import statement, the JSON file is imported as a module, and its contents are automatically parsed into a JavaScript object.
Example
// Add "type": "module" to your package.json or use .mjs extension import data from './data.json' assert { type: 'json' }; console.log(data);
Output
If you did not add "type": "module" to your package.json or use .mjs extension then this will not work.
Using the require Module
The require function is commonly used in Node.js to import modules, including JSON files. It's not available in browser environments.
When you use require to import a JSON file, Node.js automatically parses the JSON and returns it as a JavaScript object.
Example
const data = require('./data.json'); console.log(data);
Output
Conclusion
Each method of reading JSON files in JavaScript has its advantages.
- The fetch() method is versatile and works well for both local and remote JSON files in browser environments.
- The import statement provides a modern, ES6-compliant way to import JSON, treating it as a module.
- The require function is a straightforward method in Node.js environments, automatically parsing the JSON for you.
Choose the method that best fits your project's requirements and environment. Remember to handle potential errors, especially when working with file I/O or network requests.