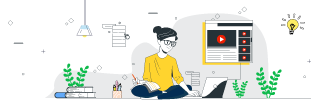
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Initialization of Local Variable in a Conditional Block in Java
Java compiler doesn’t allow abandoning an uninitialized local variable. When a local variable is initialized inside a conditional block, there are 3 possibilities that could potentially occur −
Code compiles successfully if values are provided in the conditional block and the given condition is true.
Code gives a compilation error if variables are provided (instead of values) in the conditional block and the condition is true.
Code gives compilation error if the condition that needs to be checked is false.
If the local variable is initialized to a default value outside of the conditional block in the code, it won’t give any error and the code compiles successfully.
Example
public class Demo{ public static void main(String args[]){ int i = 35; int j = 0; if (i > 32){ j = i + 11; } System.out.println("The value is: " + j); } }
Output
The value is: 46
A class named Demo contains the main function. Here, two variables are defined, and if a variable is greater than a specific number, another value is added to it and the ‘if’ block is closed. Then, the result is printed on the console.