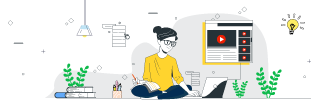
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Convert Java Util Date to SQL Date
In this article, we will learn to convert a java.util.Date object to a java.sql.Date object in Java. This is a common requirement when working with databases, as many database systems use the java.sql.Date class to represent date values. While java.util.Date is used for general date and time representation in Java, java.sql.Date is specifically designed to handle SQL-compatible date formats.
java.util.Date
The Date class in the java.util package representing a specific moment in time, measured in milliseconds since January 1, 1970, UTC (the "epoch"). It's a general-purpose date and time representation.
java.sql.Date
A subclass of java.util.Date, specifically designed for JDBC (Java Database Connectivity). It represents only the date portion (year, month, and day) without the time component.
Why This Conversion?
SQL date fields typically do not include time information. We are converting to java.sql.Date ensures compatibility and avoids runtime errors when using PreparedStatement or other database operations.
Using java.sql.Date Constructor
Below is an example to convert a java.util.Date object to a java.sql.Date object ?
import java.util.Date; public class Example { public static void main(String args[]) { // util object java.util.Date utilObj = new java.util.Date(); // sql object java.sql.Date sqlObj = new java.sql.Date(utilObj.getTime()); System.out.println("Util Date = " + utilObj); System.out.println("SQL Date = " + sqlObj); } }
Output
Util Date = Mon Nov 19 05:48:00 UTC 2018 SQL Date = 2018-11-19
Using java.sql.Date.valueOf() method
To convert from a java.util.Date object to a java.sql.Date objects, there are multiple approaches. Here's an alternate method using the java.sql.Date.valueOf() method with LocalDate from java.time package.LocalDate
The LocalDate class represents a date without time or timezone, often used for simpler date manipulation.
To convert java.util.Date to LocalDate, the toInstant() method converts the Date to an Instant, which is then associated with the system's default time zone using atZone(). The toLocalDate() method extracts the date portion as a LocalDate.
// Convert java.util.Date to LocalDate LocalDate localDate = utilDate.toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
To convert a LocalDate to java.sql.Date, the Date.valueOf() method is used, directly transforming the LocalDate into a java.sql.Date. These conversions ensure compatibility between legacy Date objects and modern LocalDate APIs.
// Convert LocalDate to java.sql.Date Date sqlDate = Date.valueOf(localDate); System.out.println("java.sql.Date: " + sqlDate);
Example
Below is an example to convert a java.util.Date object to a java.sql.Date object ?
import java.time.LocalDate; import java.time.ZoneId; public class DateConversion { public static void main(String[] args) { // Create a java.util.Date object (e.g., current date and time) java.util.Date utilDate = new java.util.Date(); System.out.println("java.util.Date: " + utilDate); // Convert java.util.Date to LocalDate LocalDate localDate = utilDate.toInstant() .atZone(ZoneId.systemDefault()) .toLocalDate(); // Convert LocalDate to java.sql.Date using valueOf method java.sql.Date sqlDate = java.sql.Date.valueOf(localDate); System.out.println("java.sql.Date: " + sqlDate); } }
Output
Util Date = Mon Nov 19 05:48:00 UTC 2018
SQL Date = 2018-11-19