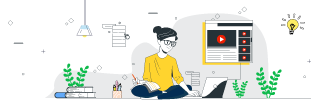
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Map Sum Pairs in JavaScript
Problem
We are required to implement a MapSum class with insert, and sum methods. For the method insert, we'll be given a pair of (string, integer). The string represents the key and the integer represents the value. If the key already existed, then the original key-value pair will be overridden to the new one.
For the method sum, we'll be given a string representing the prefix, and we need to return the sum of all the pairs' values whose key starts with the prefix.
Example
Following is the code −
class Node { constructor(val) { this.num = 0 this.val = val this.children = {} } } class MapSum { constructor(){ this.root = new Node(''); } } MapSum.prototype.insert = function (key, val) { let node = this.root for (const char of key) { if (!node.children[char]) { node.children[char] = new Node(char) } node = node.children[char] } node.num = val } MapSum.prototype.sum = function (prefix) { let sum = 0 let node = this.root for (const char of prefix) { if (!node.children[char]) { return 0 } node = node.children[char] } const helper = (node) => { sum += node.num const { children } = node Object.keys(children).forEach((key) => { helper(children[key]) }) } helper(node) return sum } const m = new MapSum(); console.log(m.insert('apple', 3)); console.log(m.sum('ap'));
Output
undefined 3
Advertisements