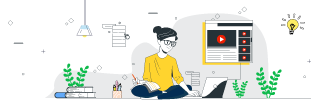
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Three-Way Partitioning of an Array Around a Given Range Using Python
Given an array and the range of the array [startval, endval]. Array is divided by three parts.
All elements smaller than startval come first.
All elements in range startval to endval come next.
All elements greater than endval appear in the end.
Let's say we have the following input ?
A = [1, 14, 51, 12, 4, 2, 54, 20, 87, 98, 3, 1, 32] startval = 14, endval = 54
The output should be ?
A = [1, 12, 4, 2, 3, 1, 14, 51, 20, 32,54, 87, 98]
Three-way partitioning of an array around a given range with List Comprehension
In this example, we will see how to three-way partition an array around a given range ?
Example
def partition_array(input, lowVal, highVal): # Separate input list in three parts my_first = [ num for num in input if num<lowVal ] my_second = [ num for num in input if (num>=lowVal and num<=highVal) ] my_third = [ num for num in input if num>highVal ] # Concatenate all the three parts print(my_first + my_second + my_third) # Driver program if __name__ == "__main__": my_input = [10, 140, 50, 200, 40, 20, 540, 200, 870, 980, 30, 10, 320] my_lowVal = 140 my_highVal = 200 partition_array(my_input, my_lowVal, my_highVal)
Output
[10, 50, 40, 20, 30, 10, 140, 200, 200, 540, 870, 980, 320]
Three-way partitioning of an array around a given range with while loop
In this example, we will see how to three-way partition an array around a given range with while loop ?
Example
def partitionFunc(my_input, n, lowVal, highVal): begn = 0 end = n - 1 i = 0 # Looping while i <= end: if my_input[i] < lowVal: my_input[i], my_input[begn] = my_input[begn], my_input[i] i += 1 begn += 1 elif my_input[i] > highVal: my_input[i], my_input[end] = my_input[end], my_input[i] end -= 1 else: i+=1 # Driver code if __name__ == "__main__": my_input = [1, 14, 51, 12, 4, 2, 54, 20, 87, 98, 3, 1, 32] n = len(my_input) partitionFunc(my_input, n, 14, 54) for i in range(n): print(my_input[i], end = " ")
Output
1 12 4 2 1 3 54 20 32 51 14 98 87
Advertisements