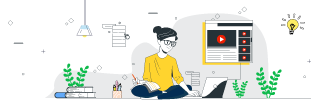
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Virtual Functions and Runtime Polymorphism in C++
In C++, both virtual functions and runtime polymorphism are key features that enable dynamic behavior and code flexibility.
Virtual Functions
A virtual function is the member function that is declared in the base class using the keyword virtual and is overridden in the derived class.
The virtual function enables runtime polymorphism means the function that will be executed is determined at runtime rather than at compile time.
Characteristics of Virtual Function
Following are the characteristics of the virtual function:
- Virtual function make sure the correct function is called for an object, regardless of the type of the reference (or pointer) for the function call.
- They are mainly used to achieve runtime polymorphism and resolve the function call at runtime.
Syntax
Following is the syntax of the virtual function:
class Base{ public: virtual return_type functionName(){ .... } } class Derived : public Base{ public: // Overriden virtual function // of the base class return_type functionName(){ ... } }
Example: Implementation of Virtual Function
Let's implement the virtual function in the following C++ example:
#include <iostream> using namespace std; class Base { public: // Virtual function virtual void display() { cout << "Base class display function" << endl; } }; class Derived: public Base { public: void display() { cout << "Derived class display function" << endl; } }; int main() { Base * ptr; Derived obj; ptr = & obj; // Calling the virtual function ptr -> display(); return 0; }
Runtime Polymorphism
The term polymorphism means the ability to make many forms occurs if there is a hierarchy of classes that are related to each other by inheritance.
Runtime Polymorphism: It is also known as dynamic polymorphism. Function overriding is an example of runtime polymorphism. The call to the function is determined at the runtime is known as runtime polymorphism.
Function Overriding
When the child class contains a method that is already present in the parent class, hence child class overrides the method of the parent class. Basically, Parents and child contains the same function with different definitions.
Characteristics of Runtime Polymorphism
Following are the characteristics of the runtime polymorphism:
- It is also known as dynamic polymorphism.
- It allows objects of different classes to be treated as instances of common parent classes.
- The runtime polymorphism is achieved by the method overriding.
Syntax
Following is the syntax of the runtime polymorphism:
class Base { public: virtual return_type function() { ... } }; class Derived : public Base { public: // Overriding the base class function return_type function() override { .... } };
Example: Implementation of The Runtime Polymorphism in C++
Following is the C++ example implementation of the runtime polymorphism we achieve runtime polymorphism using the virtual keyword:
#include <iostream> using namespace std; class Animal { public: virtual void makeSound() { cout << "Animal sound" << std::endl; } }; class Dog: public Animal { public: void makeSound() override { cout << "Woof!" << std::endl; } }; class Cat: public Animal { public: void makeSound() override { cout << "Meow!" << std::endl; } }; int main() { Animal * animal1 = new Animal(); Animal * animal2 = new Dog(); Animal * animal3 = new Cat(); animal1 -> makeSound(); animal2 -> makeSound(); animal3 -> makeSound(); return 0; }
Following is the output:
Animal sound Woof! Meow!
We can conclude that virtual function is a mechanism to achieve runtime polymorphism in object-oriented programming.