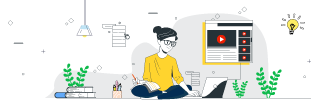
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Why C/C++ Variables Can't Start with Numbers
In C/C++, a variable name can have alphabets, numbers, and the underscore( _ ) character. There are some keywords in the C/C++ language. Apart from them, everything is treated as an identifier. Identifiers are the names of variables, constants, functions, etc.
Why Variables in C/C++ Can't Start with Numbers?
In C and C++, variable names (also, known as identifiers) cannot start with a digit due to how the compiler processes code during compilation.
First, we need to understand the phases of compilation or compiler. There are seven phases in a typical compiler:
- Lexical Analysis
- Syntax Analysis
- Semantic Analysis
- Intermediate Code Generation
- Code Optimization
- Code Generation
- Symbol Table Management
The Lexical Analysis phase is responsible for reading the source code and breaking it into tokens (like identifiers, keywords, literals, etc.).
Why Starting a Variable with a Number Is Invalid?
We can not specify the identifier that starts with a number in C/C++ because:
- None of the above supports that a variable starts with a number.
- Suppose that a variable starts with a number. First, it will scan the numbers, and then scan the alphabet later on.
- When it finds the alphabet after the numbers, it gets confused.
- So the compiler will have to backtrack to the lexical analysis phase, but backtracking is not supported in most compilers.
- The compiler should be able to identify a token as an identifier or a literal after looking at the first character.
Examples Demonstrating Why Variables Can't Start with Numbers
Practice these examples to understand variable (identifier) naming conventions, especially why variables cannot start with a number.
Example 1
In this example, we are initializing three variables. Out of three variables, one variable's name starts with a number. We will get an error when we run the code in C and C++.
#include <stdio.h> int main() { int 5s = 8; int _4a = 3; int b = 12; printf("The value of variable 5s : %d", 5s); printf("The value of variable _4a : %d", _4a); printf("\nThe value of variable b : %d", b); return 0; }
The output of the above code is as follows:
This will throw an error: error: invalid suffix "s" on integer constant
#include <iostream> using namespace std; int main() { int 5s = 8; int _4a = 3; int b = 12; cout << "The value of variable 5s :" << 5s; cout << "The value of variable _4a :" << _4a; cout << "\nThe value of variable b :" << b; return 0; }
The output of the above code is as follows:
This will throw an error: error: expected unqualified-id before numeric constant
Example 2
In the example given below, we have used two variables where one variable starts with an underscore (_) and the other variable starts with an alphabet. This will not give any error as both are valid ways to define variables.
#include <stdio.h> int main() { int _4a = 3; int b = 12; printf("The value of variable _4a : %d", _4a); printf("\nThe value of variable b : %d", b); return 0; }
The output of the above code is as follows:
The value of variable _4a : 3 The value of variable b : 12
#include <iostream> using namespace std; int main() { int _4a = 3; int b = 12; cout << "The value of variable _4a :" << _4a; cout << "\nThe value of variable b :" << b; return 0; }
The output of the above code is as follows:
The value of variable _4a :3 The value of variable b :12