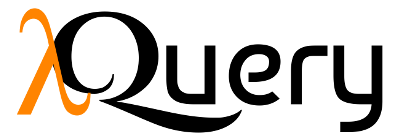
lquery
3.2.0A library to allow jQuery-like HTML/DOM manipulation.
About lQuery
lQuery is a DOM manipulation library written in Common Lisp, inspired by and based on the jQuery syntax and functions. It uses Plump and CLSS as DOM and selector engines. The main idea behind lQuery is to provide a simple interface for crawling and modifying HTML sites, as well as to allow for an alternative approach to templating.
How To
Load lQuery with ASDF or Quicklisp.
(ql:quickload :lquery)
First, lQuery needs to be initialized with a document to work on:
(defvar *doc* (lquery:$ (initialize (asdf:system-relative-pathname :lquery "test.html"))))
After that, you can use the $
macro to select and manipulate the DOM:
(lquery:$ *doc* "article")
(lquery:$ *doc*
"article"
(add-class "fancy")
(attr "foo" "bar"))
To render the HTML to a string use SERIALIZE
. If you want to save it to a file directly, there's also WRITE-TO-FILE
.
(lquery:$ *doc* (serialize))
(lquery:$ *doc* (write-to-file #p"~/plump-test.html"))
So a quick file manipulation could look something like this:
(lquery:$ (initialize (asdf:system-relative-pathname :lquery "test.html"))
"article"
(append-to "#target")
(add-class "foo")
(root)
(write-to-file #p"~/plump-test.html"))
Aside from using selectors as the first step, it's also possible to use any other variable or list and operate on it.
Since 2.0: Literal function calls need to be added with INLINE
.
Note that the result of the inline function will be used as if literally put in place. For example, an inlined function that evaluates
to a string will result in a CSS-select.
(lquery:$ (inline (list node-a node-b))
"article"
(serialize))
Selectors can come at any point in the sequence of lQuery operations and will always act on the current set of elements. If an operation evaluates to a list, array, vector or a single node, the current set of elements is set to this result.
(lquery:$ *doc*
"a"
(text "Link")
(inline (lquery:$ *doc* "p"))
(text "Paragraph"))
This is equivalent to the following:
(lquery:$ *doc*
"a" (text "Link"))
(lquery:$ *doc*
"p" (text "Paragraph"))
Functions in the argument list will be translated to a function invocation with the current list of elements as their argument.
(lquery:$ *doc*
"a" #'(lambda (els) (aref els 0)))
lQuery2.0 also supports compile-time evaluation of forms, whose results are then put in place of their function calls:
(lquery:$ *doc* (eval (format NIL "~a" *selector*)))
Keep in mind that the lexical environment is not the same at compile-time as at run-time.
Often times you'll also want to retrieve multiple, different values from your current set of nodes. To make this more convenient, you can use the COMBINE
form:
(lquery:$ *doc*
"a"
(combine (attr :href) (text))
(map-apply #'(lambda (url text) (format T "[~a](~a)" text url))))
lQuery uses vectors internally to modify and handle sets of nodes. These vectors are usually modified instead of copied to avoid unnecessary resource allocation. This however also means that lQuery functions are possibly side-effecting. If you pass an adjustable vector into lQuery through INLINE
or similar, it will not be copied and therefore side-effects might occur. lQuery will automatically copy everything else that isn't an adjustable vector through ENSURE-PROPER-VECTOR
. If you do want to pass in an adjustable vector, but make sure it doesn't affect it, use COPY-PROPER-VECTOR
.
Test Suite
To ensure that functions are at least somewhat stable in their behaviour, lQuery includes a test suite. You can load this through Quicklisp/ASDF with
(ql:quickload :lquery-test)
(lquery-test:run)
The tests are rather loose, but should cover all functions to at least behave mostly according to expectation.
Extending lQuery3.1
lQuery allows extension in a couple of ways. The most important of which are node functions themselves, which come in two flavours: lquery-funs and lquery-list-funs.
Any lquery function resides in the package LQUERY-FUNCS
, which is automatically scanned by the $ macro. The two macros responsible for defining new lquery functions automatically place the resulting operations in this package for you.
(define-lquery-function name (node-name &rest arguments) &body body)
(define-lquery-list-function name (vector-name &rest arguments) &body body)
Any function generated by these macros can be called either with a single node or a vector of nodes. In the case of a regular node operation, if it receives a vector of nodes, the function is called once for each node and the results are collected into a vector, which is then returned. If it receives a single node, only a single result is returned. In the case of a node list function, the return value can be either a vector or a single value, depending on what the goal of the operation is. It is expected that node list functions will modify the given vector directly in order to avoid unnecessary copying.
Some constructs would be very cumbersome to write as functions, or would simply be more suited in the form of a macro. To allow for this, lQuery3.1 includes a mechanism of $
local macros. The previously mentioned forms like INLINE
are handled through this system. Just like lquery functions, lquery macros reside in their own package LQUERY-MACROS
. The responsible macro for defining new lquery macros will automatically place it in there for you.
(define-lquery-macro name (previous-form &rest arguments) &body body)
The $ macro itself can be extended as well by providing additional argument- or value-handlers. The following two macros make this possible:
(define-argument-handler type (argument-name operator-name) &body body)
Argument handlers transform the arguments at compile-time. For example, this would allow an extension to turn literal arrays into lists so they can be processed as well.
(define-value-handler type (variable-name operator-name) &body body)
Value handlers on the other hand determine the action at run-time. This is mostly useful for defining special actions on certain variable values.
What's New
3.1.0
Renamed DEFINE-NODE-\*
macros into more sensible DEFINE-LQUERY-*
.
Added macro system, new standard COMBINE
macro.
3.0.0
Complete rewrite of everything. This version is compatibility breaking. While the node functions themselves perform just the same as before (with one or two exceptions), lQuery now uses vectors instead of lists internally. If you ever relied on lQuery return values, this version will most likely break your code. Effort has been made to keep upgrading as simple as possible though; passing lists into an lQuery chain automatically transforms it for example.
Thanks to the change to Plump, lQuery is now also able to parse almost any kind of X/HT/ML document, which was not well possible previously. And thanks to switching to CLSS, lQuery is now much faster at selecting nodes from the DOM.
2.0.0
Added extension system and INLINE
, EVAL
handling. Revamped base macros to be more stable and simple.
Further Reading
System Information
Definition Index
-
LQUERY
- ORG.SHIRAKUMO.LQUERY
No documentation provided.-
EXTERNAL SPECIAL-VARIABLE *LQUERY-MASTER-DOCUMENT*
The master document used at the beginning of a chain.
-
EXTERNAL FUNCTION COPY-PROPER-VECTOR
- SEQUENCE
- &KEY
- (TRANSFORM #'IDENTITY)
Copies the sequence into a new proper vector.
-
EXTERNAL FUNCTION ENSURE-PROPER-VECTOR
- VAR
Ensure that the variable is a proper vector.
-
EXTERNAL FUNCTION EVAL
- ORIGINAL-EXP
Evaluate the argument in a null lexical environment, returning the result or results.
-
EXTERNAL FUNCTION INITIALIZE
- DOCUMENT
Sets the *lquery-master-document* variable to the provided document.
-
EXTERNAL FUNCTION LOAD-PAGE
- FILE-OR-STRING
Load the given file or string into a HTML DOM.
-
EXTERNAL FUNCTION MAKE-PROPER-VECTOR
- &KEY
- (SIZE 0)
- INITIAL-ELEMENT
- INITIAL-CONTENTS
- (FILL-POINTER T)
Creates a new proper vector.
-
EXTERNAL FUNCTION PARSE-HTML
- HTML
Build the given string into DOM objects related to the master document.
-
EXTERNAL MACRO $
- &BODY
- ACTIONS
Performs lQuery operations on the current document. Each argument is executed in sequence. The arguments are evaluated according to the defined argument-handlers. By default, the following cases are handled: * STRING Translates to a CLSS:QUERY on the current elements. * FUNCTION Translates to a function call with the list of nodes as argument. * SYMBOL Delegates to the value handlers. * LIST Lists are transformed according to their first element, which must be a symbol. If the symbol's name corresponds to a function found in the LQUERY-MACROS package, The form is assembled according to that function. Otherwise if it corresponds to an LQUERY-FUNCS function, it is expanded into a call to that function. If the symbol cannot be found in either package, it is put back in place, but the call itself is assembled like so: (FUNCTION PREVIOUS-RESULT ARGUMENT*) Values are handled at runtime according to the defined variable-handlers. By default, the following cases are handled at run time: * STRING Performs a CLSS:QUERY on the current elements. * DOM:NODE Replaces the current set of nodes with just this node. * FUNCTION Calls the given function with the current set of nodes as argument. * LIST Lists are transformed into a proper vector. * ARRAY Arrays are transformed into a proper vector. * VECTOR Vectors that are not adjustable are transformed into a proper vector. * T Any other value simply replaces the current list of nodes.
-
EXTERNAL MACRO $1
- &BODY
- ACTIONS
This is the same as $, except it automatically uses NODE at the end and thus only returns the first result, if any.
-
EXTERNAL MACRO DEFINE-ARGUMENT-HANDLER
- TYPE
- (ARGUMENT-NAME OPERATOR-NAME)
- &BODY
- BODY
Defines a new argument handler that decides what to do with a certain type of argument at compile-time. TYPE --- A type or EQL specifier. ARGUMENT-NAME --- Symbol bound to the argument. OPERATOR-NAME --- Symbol bound to the object being operated on. BODY ::= form*
-
EXTERNAL MACRO DEFINE-LQUERY-FUNCTION
- NAME
- (NODE-NAME &REST ARGUMENTS)
- &BODY
- BODY
Defines a new node function. This is the main mechanism by which node manipulations are defined. All lquery functions are automatically created in the lquery-funcs package. NAME --- A symbol naming the lquery function. Automatically interned in the LQUERY-FUNCS package. NODE-NAME --- Symbol bound to the current node. ARGUMENTS --- A lambda-list specifying the arguments for the function. BODY ::= form*
-
EXTERNAL MACRO DEFINE-LQUERY-LIST-FUNCTION
- NAME
- (VECTOR-NAME &REST ARGUMENTS)
- &BODY
- BODY
Defines a new function that operates on the current node array instead of individual elements. All lquery functions are automatically created in the lquery-funcs package. NAME --- A symbol naming the lquery function. Automatically interned in the LQUERY-FUNCS package. VECTOR-NAME --- Symbol bound to the node vector. ARGUMENTS --- A lambda-list specifying the arguments for the function. BODY ::= form*
-
EXTERNAL MACRO DEFINE-LQUERY-MACRO
- NAME
- (PREVIOUS-FORM &REST ARGUMENTS)
- &BODY
- BODY
Define a new lquery local macro. All lquery macros are automatically created in the lquery-macros package. NAME --- A symbol naming the lquery macro. Automatically interned in the LQUERY-MACROS package. PREVIOUS-FORM --- Symbol bound to the so far assembled form, the previous value so to speak. ARGUMENTS --- A lambda-list specifying the arguments for the macro (note that this must be a standard lambda-list). BODY ::= form*
-
EXTERNAL MACRO DEFINE-LQUERY-SUBROUTINE
- NAME
- (&REST ARGUMENTS)
- &BODY
- BODY
Defines a shorthand function. The body is a set of lQuery instructions as you'd use in $. NAME --- A symbol naming the subroutine. Automatically interned in the LQUERY-FUNCS package. ARGUMENTS --- A lambda-list specifying the arguments for the function. BODY ::= lquery-form*
-
EXTERNAL MACRO DEFINE-VALUE-HANDLER
- TYPE
- (VARIABLE-NAME OPERATOR-NAME)
- &BODY
- BODY
Defines a new symbol handler that decides what to do with a certain type of symbol at run-time (variable type). TYPE --- A type or EQL specifier. VARIABLE-NAME --- Symbol bound to the argument. OPERATOR-NAME --- Symbol bound to the object being operated on. BODY ::= form*
-
EXTERNAL MACRO WITH-MASTER-DOCUMENT
- (&OPTIONAL (DOC))
- &BODY
- BODY
Surrounds the body in a binding for the *lquery-master-document* to ensure it does not get clobbered.
-
LQUERY-MACROS
- ORG.SHIRAKUMO.LQUERY.MACROS
No documentation provided.-
EXTERNAL FUNCTION COMBINE
- NODES
- &REST
- CALLS
COMBINES multiple lquery function calls into one by gathering them into a list for each element. ($ (combine (text) (attr :a))) would be equivalent to ($ (map #'(lambda (node) (list (lquery-funcs:text node) (lquery-funcs:attr node :a))))) This construct is especially useful in combination with MAP-APPLY.
-
EXTERNAL FUNCTION EVAL
- NODES
- FORM
Evaluates the form at compile-time and puts its resulting value in place.
-
EXTERNAL FUNCTION FUNCTION
- NODES
- NAME
Macro to allow #'foo to be used in lquery chains.
-
EXTERNAL FUNCTION INITIALIZE
- NODES
- &REST
- INIT-CALLS
See lquery function INITIALIZE. This is merely a performance macro to avoid the unnecessary default allocation of a vector.
-
EXTERNAL FUNCTION INLINE
- NODES
- FORM
Treats the form as if the evaluated value was put literally in place. See DETERMINE-VALUE.
-
LQUERY-FUNCS
- ORG.SHIRAKUMO.LQUERY.FUNCS
No documentation provided.-
EXTERNAL FUNCTION ADD
- WORKING-NODES
- SELECTOR-OR-NODES
Add elements to the set of matched elements.
-
EXTERNAL FUNCTION ADD-CLASS
- NODE
- &REST
- CLASSES
Adds the specified class(es) to the set of matched elements.
-
EXTERNAL FUNCTION AFTER
- NODES
- HTML-OR-NODES
Insert content (in html-string or node-list form) after each element.
-
EXTERNAL FUNCTION ANCESTOR
- WORKING-NODES
Find the common ancestor of all elements.
-
EXTERNAL FUNCTION APPEND
- NODES
- HTML-OR-NODES
Insert content (in html-string or node-list form) to the end of each element.
-
EXTERNAL FUNCTION APPEND-TO
- WORKING-NODES
- SELECTOR-OR-NODES
Insert every element to the end of the target(s).
-
EXTERNAL FUNCTION ATTR
- NODE
- &REST
- PAIRS
Retrieve or set attributes on a node. The value on a node is turned into a string using PRINC-TO-STRING. If a value is NIL, the associated attribute is removed.
-
EXTERNAL FUNCTION BEFORE
- NODES
- HTML-OR-NODES
Insert content (in html-string or node-list form) before each element.
-
EXTERNAL FUNCTION CHILD-INDEX
- NODE
Returns the index of the element within its parent, also counting text nodes. See index() otherwise.
-
EXTERNAL FUNCTION CHILDREN
- NODES
- &OPTIONAL
- SELECTOR
Get the children of each element, optionally filtered by a selector.
-
EXTERNAL FUNCTION CLONE
- NODE
Create a deep copy of the set of matched elements.
-
EXTERNAL FUNCTION CLOSEST
- NODE
- SELECTOR
For each element in the set, get the first element that matches the selector by testing the element itself and traversing up through its ancestors in the DOM tree. If no matching element can be found the root is entered instead.
-
EXTERNAL FUNCTION CONTAINS
- NODES
- STRING
Select all elements that contain the specified text.
-
EXTERNAL FUNCTION CONTENTS
- NODES
Get the children of each element, including text and comment nodes.
-
EXTERNAL FUNCTION CSS
- NODE
- &REST
- PAIRS
Retrieve or set css style attributes on a node.
-
EXTERNAL FUNCTION DATA
- NODE
- &REST
- PAIRS
Retrieve or set data attributes on a node. This is a convenience method and uses attr in the back.
-
EXTERNAL FUNCTION DEEPEST
- NODE
Returns the innermost (left-bound) child element.
-
EXTERNAL FUNCTION DETACH
- NODES
- &OPTIONAL
- SELECTOR
Removes the node (optionally filtered by the selector) from the document. Alias for remove()
-
EXTERNAL FUNCTION EACH
- NODES
- FUN
- &KEY
- REPLACE
Execute the specified function on each element until NIL is returned or all elements have been processed. The original set of elements is returned if replace is NIL.
-
EXTERNAL FUNCTION EMPTY
- NODE
Remove all child nodes from the set of matched elements.
-
EXTERNAL FUNCTION EMPTY-P
- NODE
Check if the node contains no children and/or only empty (whitespace) text nodes. If it is empty, T is returned, otherwise NIL.
-
EXTERNAL FUNCTION EQ
- WORKING-NODES
- INDEX
Reduce the set of matched elements to the one at the specified index
-
EXTERNAL FUNCTION EVEN
- WORKING-NODES
Selects even elements, 1-indexed
-
EXTERNAL FUNCTION FILTER
- NODES
- SELECTOR-OR-FUNCTION
Reduce the set of matched elements to those that match the selector or pass the function's test.
-
EXTERNAL FUNCTION FIND
- NODES
- SELECTOR-OR-FUNCTION
- &KEY
- (TEST-SELF NIL)
Get the descendants of each element filtered by selector or function.
-
EXTERNAL FUNCTION FIRST
- WORKING-NODES
Reduce the set of matched elements to the first in the set.
-
EXTERNAL FUNCTION GT
- WORKING-NODES
- INDEX
Select all elements at a greater than index(0) within the matched set.
-
EXTERNAL FUNCTION HAS
- NODES
- SELECTOR-OR-NODES
Reduce the set of matched elements to those that have a descendant that matches the selector or element.
-
EXTERNAL FUNCTION HAS-CLASS
- WORKING-NODES
- CLASS
Determine whether any of the matched elements are assigned to the given class.
-
EXTERNAL FUNCTION HIDE
- WORKING-NODES
Hide the matched elements (short for (css "display" "none")).
-
EXTERNAL FUNCTION HTML
- NODE
- &OPTIONAL
- NEW-CONTENT
Get the HTML contents of the elements or set the HTML contents of every matched element. The new content can be either a plump node, root, pathname, or string. If it is none of those, it is treated as a string via PRINC-TO-STRING
-
EXTERNAL FUNCTION HTML-FILE
- WORKING-NODES
- PATHNAME
Read an HTML file and insert its contents into each element.
-
EXTERNAL FUNCTION INDEX
- NODE
Find the index of the node within its parent.
-
EXTERNAL FUNCTION INITIALIZE
- WORKING-NODES
- DOCUMENT
Re-initializes lQuery with a new page.
-
EXTERNAL FUNCTION INSERT-AFTER
- WORKING-NODES
- SELECTOR-OR-NODES
Insert every element after the target.
-
EXTERNAL FUNCTION INSERT-BEFORE
- WORKING-NODES
- SELECTOR-OR-NODES
Insert every element before the target.
-
EXTERNAL FUNCTION IS
- WORKING-NODES
- SELECTOR-OR-NODES
Check the current elements against a selector or list of elements and return true if at least one of them matches.
-
EXTERNAL FUNCTION IS-EMPTY
- NODE
Check if the node contains no children and/or only empty (whitespace) text nodes. If it is empty, T is returned, otherwise NIL. Alias of EMPTY-P
-
EXTERNAL FUNCTION LAST
- WORKING-NODES
Reduce the set of matched elements to the final one in the set.
-
EXTERNAL FUNCTION LENGTH
- WORKING-NODES
Returns the number of elements in the list.
-
EXTERNAL FUNCTION LT
- WORKING-NODES
- INDEX
Select all elements at an index less than the index within the matched set.
-
EXTERNAL FUNCTION MAP
- WORKING-NODES
- FUNCTION
Pass each element through a function (which has to accept one argument, the node), returning the list of all results.
-
EXTERNAL FUNCTION MAP-APPLY
- WORKING-NODES
- FUNCTION
Pass each element through a function by apply, returning the vector of all results. This is commonly useful in combination with COMBINE.
-
EXTERNAL FUNCTION NEXT
- NODES
- &OPTIONAL
- SELECTOR
Get the immediately following sibling of each element (if there is one). If a selector is provided, the sibling is only included if it matches.
-
EXTERNAL FUNCTION NEXT-ALL
- NODES
- &OPTIONAL
- SELECTOR
Get all following siblings of each element. If a selector is provided, the sibling is only included if it matches.
-
EXTERNAL FUNCTION NEXT-UNTIL
- NODES
- SELECTOR-OR-NODES
Get all following silings of each element up to (excluding) the element matched by the selector or node list.
-
EXTERNAL FUNCTION NODE
- WORKING-NODES
- &OPTIONAL
- (N 0)
Return the specified node (default first) directly, without encompassing it into a vector if it exists. Otherwise return NIL.
-
EXTERNAL FUNCTION NOT
- WORKING-NODES
- SELECTOR-OR-NODES
Remove matching elements from the working elements.
-
EXTERNAL FUNCTION NOT-EMPTY
- NODE
Check if the node contains no children and/or only empty (whitespace) text nodes. If the node is effectively empty NIL is returned, otherwise T
-
EXTERNAL FUNCTION ODD
- WORKING-NODES
Select all odd elements from the current set, 1-indexed.
-
EXTERNAL FUNCTION PARENT
- NODES
- &OPTIONAL
- SELECTOR
Get the parent of each element, optionally filtered by a selector.
-
EXTERNAL FUNCTION PARENTS
- NODES
- &OPTIONAL
- SELECTOR
Get the ancestors of each element, optionally filtered by a selector. Closest parent first.
-
EXTERNAL FUNCTION PARENTS-UNTIL
- NODES
- SELECTOR-OR-NODES
Get the ancestors of each element, up to (excluding) the element matched by the selector or node list. Closest parent first
-
EXTERNAL FUNCTION PREPEND
- NODES
- HTML-OR-NODES
Insert content, specified by the parameter, to the beginning of each element.
-
EXTERNAL FUNCTION PREPEND-TO
- WORKING-NODES
- SELECTOR-OR-NODES
Insert every element to the beginning of the target(s).
-
EXTERNAL FUNCTION PREV
- NODES
- &OPTIONAL
- SELECTOR
Get the immediately preceding sibling of each element (if there is one). If a selector is provided, the sibling is only included if it matches.
-
EXTERNAL FUNCTION PREV-ALL
- NODES
- &OPTIONAL
- SELECTOR
Get all preceeding siblings of each element. If a selector is provided, the sibling is only included if it matches.
-
EXTERNAL FUNCTION PREV-UNTIL
- NODES
- SELECTOR-OR-NODES
Get all preceeding silings of each element down to (excluding) the element matched by the selector or node list.
-
EXTERNAL FUNCTION REMOVE
- NODE
- &OPTIONAL
- SELECTOR
Remove the set of matched elements from the DOM.
-
EXTERNAL FUNCTION REMOVE-ATTR
- NODE
- &REST
- ATTRIBUTES
Remove attributes from each element.
-
EXTERNAL FUNCTION REMOVE-CLASS
- NODE
- &REST
- CLASSES
Remove classes from each element.
-
EXTERNAL FUNCTION REMOVE-DATA
- NODE
- &REST
- DATA
Remove data attributes from each element. This is a convenience method and uses remove-attr in the back.
-
EXTERNAL FUNCTION RENDER-TEXT
- NODE
Return the "rendered" representation of the text inside the node and its children. In effect the text is gathered from the component and all of its children, but transforming the text in such a way that: - All ASCII white space (Space, Tab, CR, LF) is converted into spaces. - There are no consecutive spaces. - There are no spaces at the beginning or end.
-
EXTERNAL FUNCTION REPLACE-ALL
- WORKING-NODES
- SELECTOR-OR-NODES
Replace each in the set of matched elements with the current nodes.
-
EXTERNAL FUNCTION REPLACE-WITH
- WORKING-NODES
- HTML-OR-NODES
Replace each element with the provided new content and return the set of elements that was removed.
-
EXTERNAL FUNCTION ROOT
- WORKING-NODES
Returns to the root. Essentially traverses up the tree of the first element in the set until the root is reached.
-
EXTERNAL FUNCTION SERIALIZE
- NODE
- &OPTIONAL
- (STREAM NIL)
- (FORMAT DEFAULT)
Serialize the node into a string. Allows two optional arguments: STREAM --- NIL to return a string, or a stream to output to. FORMAT --- One of :DEFAULT, :HTML, :XML to designate the way in which to invoke Plump's serializer.
-
EXTERNAL FUNCTION SHOW
- WORKING-NODES
Display the matched elements (short for (css :display 'block'))
-
EXTERNAL FUNCTION SIBLINGS
- NODES
- &OPTIONAL
- SELECTOR
Get the siblings of each element, optionally filtered by a selector.
-
EXTERNAL FUNCTION SIZE
- WORKING-NODES
Return the number of elements in the list.
-
EXTERNAL FUNCTION SLICE
- WORKING-NODES
- START
- &OPTIONAL
- END
Reduce the set of matched elements to a subset specified by a range of indices
-
EXTERNAL FUNCTION SPLICE
- NODE
Splice the element's contents in place of itself.
-
EXTERNAL FUNCTION TEXT
- NODE
- &OPTIONAL
- (TEXT NIL T-S-P)
Get the combined text contents of each element, including their descendants. If text is set, all text nodes are removed and a new text node is appended to the end of the node. If text is NIL, all direct text nodes are removed from the node. If text is not a string, it is transformed into one by PRINC-TO-STRING.
-
EXTERNAL FUNCTION TOGGLE-CLASS
- NODE
- &REST
- CLASSES
Add or remove one or more classes from each element, depending on their presence within the element.
-
EXTERNAL FUNCTION UNWRAP
- NODE
Remove the parents of the set of matched elements from the DOM, inserting the parents children in place of it.
-
EXTERNAL FUNCTION VAL
- NODE
- &OPTIONAL
- (VALUE NIL V-P)
Get the current values or set the value of every matched element.
-
EXTERNAL FUNCTION WRAP
- NODES
- HTML-OR-NODES
Wrap an HTML structure around each element. Note that always the first node of the structure to wrap is chosen.
-
EXTERNAL FUNCTION WRAP-ALL
- WORKING-NODES
- HTML-OR-NODES
Wrap an HTML structure around all elements and put it in place of the first element, removing all other elements from their position.
-
EXTERNAL FUNCTION WRAP-INNER
- NODES
- HTML-OR-NODES
Wrap an HTML structure around the contents of each element.
-
EXTERNAL FUNCTION WRITE-TO-FILE
- WORKING-NODES
- FILE
- &KEY
- (IF-DOES-NOT-EXIST CREATE)
- (IF-EXISTS SUPERSEDE)
Write the serialized node to the file. Note that always only the first element is written.