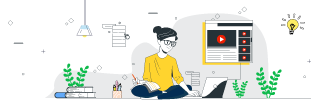
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Postorder Non-Recursive Traversal of a Given Binary Tree in C++
Binary Tree traversal is a process of visiting all the nodes in a certain order. In this article, we will learn how to perform postorder non-recursive traversal of a binary tree using two stacks in C++.
What is Postorder Non-Recursive Traversal?
Postorder traversal is a type of depth-first traversal where we first visit the left subtree, then the right subtree, and then the root node. In a non-recursive approach, we are not allowed to use recursive functions to track nodes for traversing the tree. Instead, we can use stack data structures to manually keep track of the nodes. This approach is useful in cases where recursion is not allowed or where stack overflow error needs to be avoided.
For example, for a binary tree like below:
1 / \ 2 3 / \ 4 5
The postorder traversal will be: 4 5 2 3 1
Implement Postorder Non-Recursive Traversal in C++
To implement postorder traversal without recursion, we use two stack data structures to mimick the system's recursion call stack. Below are some key points that will make you understand the method:
- Structure: Each node contains a data value and two pointers to the left and right child.
- Logic: Push root to the first stack, then move nodes from the first to the second stack while pushing left and right children. Finally, pop from the second stack to print the postorder traversal.
Steps to Perform Postorder Non-Recursive Traversal
Following are steps/algorithm to perform postorder traversal without recursion:
- Create a binary tree node structure with data, left, and right pointers.
- Initialise two empty stacks: one for processing and one for storing the result.
- Push the root node to the first stack.
- Loop while the first stack is not empty.
- Pop a node from the first stack and push it to the second stack.
- Push the left and right children of the popped node into the first stack.
- After the loop ends, pop all nodes from the second stack and print them.
C++ Program to Perform Postorder Non-Recursive Traversal
In the below code, we have implemented a binary tree and used two stacks to perform postorder traversal without recursion.
#include <iostream> #include <stack> using namespace std; // Define a node of the binary tree struct Node { int data; Node* left; Node* right; Node(int val) { data = val; left = right = nullptr; } }; // Function to perform postorder non-recursive traversal void postorderTraversal(Node* root) { if (root == nullptr) return; stack<Node*> s1, s2; s1.push(root); while (!s1.empty()) { Node* current = s1.top(); s1.pop(); s2.push(current); if (current->left) s1.push(current->left); if (current->right) s1.push(current->right); } while (!s2.empty()) { cout << s2.top()->data << " "; s2.pop(); } } int main() { // Creating tree nodes Node* root = new Node(1); root->left = new Node(2); root->right = new Node(3); root->left->left = new Node(4); root->left->right = new Node(5); cout << "Postorder Traversal (Non-Recursive): "; postorderTraversal(root); return 0; }
The output of above code will be:
Postorder Traversal (Non-Recursive): 4 5 2 3 1
Time and Space Complexity
Time Complexity: O(n), where n is the number of nodes in the binary tree.
Space Complexity: O(n), for using two stacks to hold all nodes.