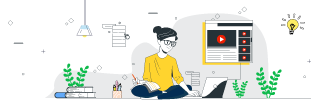
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Preorder Non-Recursive Traversal of a Given Binary Tree in C++
Tree traversal is a form of graph traversal. It involves checking or printing each node in the tree exactly once. The preorder traversal of a binary search tree involves visiting each of the nodes in the tree in the order (Root, Left, Right).
An example of Preorder traversal of a binary tree is as follows.

Here, we start at the root(3), then go to the left child (6), then its left(5), then right(2), then come back and move to the right subtree of 3 that is (4), then 9, and finally 8.
Non-Recursive Preorder Traversal of a Binary Tree
Since recursion uses the call stack to remember the current node so that after completing the left subtree we can go to the right subtree, we can manually use a stack data structure to do the same and traverse the tree without recursion.
Here's how we handle it in the program:
- We first check if the root is null. If the tree is empty, we return immediately.
- We create an empty stack and push the root node onto it.
- Then, we start a loop that runs as long as the stack is not empty:
- We take the node from the top of the stack, print its value (this is our current node).
- If the current node has a right child, we push it onto the stack.
- If it has a left child, we push that onto the stack too.
- We push the right child first so that the left child is on top and gets processed next. This maintains the correct preorder sequence: root -> left -> right.
We keep repeating this process until the stack becomes empty and all nodes are visited.
C++ Program to Perform Preorder Non-Recursive Traversal of a Given Binary Tree
Here's a complete C++ program to perform preorder non-recursive traversal:
#include <iostream> #include <stack> using namespace std; // Define structure for a tree node struct node { int data; // Value of the node struct node* left; // Pointer to left child struct node* right; // Pointer to right child }; // Function to create a new node with given value struct node* createNode(int val) { struct node* temp = new node(); // Allocate memory for new node temp->data = val; // Set node value temp->left = temp->right = nullptr; // Initialize left and right children as null return temp; } // Function to insert a value into the BST struct node* insertNode(struct node* root, int val) { if (root == nullptr) // If tree is empty, create a new node return createNode(val); if (val < root->data) // If value is smaller, go to left subtree root->left = insertNode(root->left, val); else if (val > root->data) // If value is greater, go to right subtree root->right = insertNode(root->right, val); return root; // Return unchanged root } // Iterative Preorder Traversal using Stack void preorder(struct node* root) { if (root == nullptr) // If tree is empty, return return; stack<node*> nodeStack; // Stack to hold nodes for traversal nodeStack.push(root); // Start with root node while (!nodeStack.empty()) { node* current = nodeStack.top(); // Get top node nodeStack.pop(); // Remove it from stack cout << current->data << " "; // Visit the node // Push right child first so that left child is processed first if (current->right) nodeStack.push(current->right); if (current->left) nodeStack.push(current->left); } } int main() { struct node* root = nullptr; // Initialize root node // Insert values into the BST root = insertNode(root, 5); insertNode(root, 8); insertNode(root, 3); insertNode(root, 2); insertNode(root, 6); insertNode(root, 9); insertNode(root, 4); // Perform and display preorder traversal cout << "Preorder Traversal (Non-Recursive): "; preorder(root); cout << endl; return 0; // End of program }
Once we run the above program, we'll get the following output, which shows the nodes visited in preorder:
Preorder Traversal (Non-Recursive): 5 3 2 4 8 6 9
Time Complexity: O(n) because we visit every node in the binary tree exactly once during the traversal.
Space Complexity: O(n) because in the worst case (such as a skewed tree), the stack could store up to n nodes at a time.