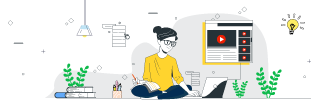
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Remove All Elements of ArrayList in Java
In Java, the ArrayList class provides various built-in methods to remove all or a single element from it.
Once those methods are called on the ArrayList, the list will become empty (). You can verify this using the size() method; it returns 0, if the list is empty.
We can remove all the elements of an ArrayList in Java:
Removing all ArrayList Elements using clear() Method
The clear() method of the List interface removes (deletes) all the elements from a list. Once this method is called on a specific list (or an ArrayList), the list will become empty ().
Following is the syntax of the clear() method:
clear()
Example
In the following example, we use the clear() method to remove all elements from the ArrayList {10, 20, 30, 40}:
import java.util.ArrayList; public class removeElements { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it, which will be remove later list.add(10); list.add(20); list.add(30); list.add(40); System.out.println("The ArrayList is: " + list); //using clear() method list.clear(); System.out.println("ArrayList after removing all elements: " + list); } }
Following is the output of the above program:
The ArrayList is: [10, 20, 30, 40] ArrayList after removing all elements: []
Using the removeAll() Method
The removeAll() method of the List interface accepts a collection as a parameter, and it removes all elements of the current list that (also) exist in the given collection.
If we use the ArrayList object, invoke this method and pass the same as a parameter to it, all the elements from the ArrayList will be removed. Following is the syntax of the removeAll() method:
boolean removeAll(Collection<?> c)
Here, c is a collection containing elements to be removed from the list.
Example
In this example, we use the removeAll() method to remove all ArrayList elements that are specified within the same list:
import java.util.ArrayList; public class removeElements { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it, which will be remove later list.add(1); list.add(2); list.add(3); list.add(4); System.out.println("The ArrayList is: " + list); //using removeAll() method to remove all ArrayList elements list.removeAll(list); System.out.println("ArrayList after removing all elements: " + list); } }
The above program produces the following output:
The ArrayList is: [1, 2, 3, 4] ArrayList after removing all elements: []