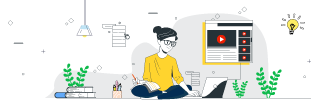
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Remove Element from ArrayList in Java
The article will discuss different ways to remove elements from an ArrayList. Below is a list of methods that can help in removing elements from an ArrayList:
- Using the remove() Method of List Interface
- Using the remove(object) Method
- Using the clear() Method
- Using the removeAll() Method
Removing a Single Element using the remove() Method
The remove() method of the List interface accepts an integer value representing an index as a parameter and removes an element at the specified index.
Following is the syntax of the remove() method:
remove(int index)
Here, the index is the position of an element in the ArrayList.
Example 1
In the following example, we use the remove() method to remove a single element at the specified index 2, in the ArrayList {10, 20, 30, 40}:
import java.util.ArrayList; public class removeElement { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it list.add(10); list.add(20); list.add(30); list.add(40); System.out.println("ArrayList before removing element: " + list); int index = 2; System.out.println("The position of an element to be removed: " + index); //using the remove() method list.remove(index); System.out.println("ArrayList after removing element: " + list); } }
The above program produces the following output:
ArrayList before removing element: [10, 20, 30, 40] The position of an element to be removed: 2 ArrayList after removing element: [10, 20, 40]
Example 2
The example below uses the remove() method inside the for-loop to remove each element one by one on every iteration from the ArrayList {10, 20, 30, 40}:
import java.util.ArrayList; public class removeElement { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it list.add(10); list.add(20); list.add(30); list.add(40); System.out.println("ArrayList before removing element: " + list); //using remove() method within for loop for(int i = 0; i<list.size(); i++){ System.out.println("Element at index " + i + " has been removed: " + list.remove(i)); //the remaining element will move the empty positions i--; } System.out.println("ArrayList after removing element: " + list); } }
Following is the output of the above program:
ArrayList before removing element: [10, 20, 30, 40] Element at index 0 has been removed: 10 Element at index 0 has been removed: 20 Element at index 0 has been removed: 30 Element at index 0 has been removed: 40 ArrayList after removing element: []
Using remove(object) Method of List Interface
The remove(object) method of the List interface accepts an object (i.e., element) as a parameter and removes the first occurrence of the specified element from the list if the element is present, or otherwise does not change.
Following is the syntax of the remove(object) method:
boolean remove(Object o)
Here, o is the element that needs to be removed if present.
Example
In the following example, we use the remove(object) method to remove the first occurrence of the specified Element "I" from the ArrayList {"A", "E", "I", "O", "U"}:
import java.util.ArrayList; public class removeElement { public static void main(String[] args) { //creating an ArrayList ArrayList<String> vowels = new ArrayList<>(); //adding element to it vowels.add("A"); vowels.add("E"); vowels.add("I"); vowels.add("O"); vowels.add("U"); System.out.println("ArrayList before removing element: " + vowels); String element = "I"; System.out.println("The element to be removed: " + element); //using the remove(object) method vowels.remove(element); System.out.println("ArrayList after removing element: " + vowels); } }
Following is the output of the above program:
ArrayList before removing element: [A, E, I, O, U] The element to be removed: I ArrayList after removing element: [A, E, O, U]
Removing all Elements using clear() Method
The clear() method of the List interface removes (deletes) all the elements from a list. Once this method is called on a specific list (or an ArrayList), the list will become empty (). Following is the syntax of the clear() method:
clear()
Example
In this example, we use the clear() method to remove all elements from the ArrayList {1, 2, 3, 4, 5}:
import java.util.ArrayList; public class removeElement { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it, which will be remove later list.add(1); list.add(2); list.add(3); list.add(4); list.add(5); System.out.println("ArrayList before removing element: " + list); //using clear() method list.clear(); System.out.println("ArrayList after removing all elements: " + list); } }
Below is the output of the above program:
ArrayList before removing element: [1, 2, 3, 4, 5] ArrayList after removing all elements: []
Using removeAll() Method of List Interface
The removeAll() method of the List interface accepts a collection as a parameter, and removes all elements from the current list object that exist in the given collection.
To remove all the elements from an ArrayList object, we need to invoke this method on it by passing the same as a parameter.
Following is the syntax of the removeAll() method:
boolean removeAll(Collection<?> c)
Here, c is a collection containing elements to be removed from the list.
Example
In the following example, we use the removeAll() method to remove all ArrayList elements:
import java.util.ArrayList; public class removeElements { public static void main(String[] args) { //creating an ArrayList ArrayList<Integer> list = new ArrayList<>(); //adding element to it, which will be remove later list.add(1); list.add(2); list.add(3); list.add(4); System.out.println("The ArrayList is: " + list); //using removeAll() method to remove all ArrayList elements list.removeAll(list); System.out.println("ArrayList after removing all elements: " + list); } }
The above program produces the following output:
The ArrayList is: [1, 2, 3, 4] ArrayList after removing all elements: []