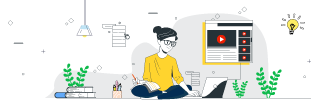
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Why Array Index Starts from Zero in C/C++
An array arr[i] is interpreted as *(arr+i). Here, arr denotes the address of the first array element or the 0th index element. So *(arr+i) means the element is at i distance from the first element of the array. So, array index starts from 0 as initially i is 0 which means the first element of the array.
In this article, we will see an example of C and C++ code to understand the reason why an array starts from index 0.
Why Array Index Starts from Zero?
The array index starts from zero as it provides better efficiency and less calculation during calculating memory address for storing the array elements. The languages such as C and C++ uses contiguous memory allocation using row-major order. When index starts with 0, the first element maps with base address automatically. Here is the formulas to calculate the address of the element when index starts from '0' and when it starts from '1'.
Formula to Calculate Memory Address of Array Elements
The formula for calculating the memory address of array elements when the index starts from '0' and '1' is mentioned below:
When index starts with i=0 Address(arr[i]) = Base address + i * size(data type) For example, if base address is 2000, and size = 4 Address will be: 2000 + 0 *4 = 2000 When index starts with i=1 Address(arr[i]) = Base address + (i-1) * size(data type) For example, if base address is 2000, and size = 4 Address will be: 2000 + (1-1) *4 = 2000
In the above formula, we can see that we need an extra subtraction operation for address calculation when the index starts with '1'.
Example
Here is an example of C and C++ code where we have printed the element at '0th' and '1st' position along with their pointer and memory address:
#include <stdio.h> int main() { int arr[] = {100, 200, 300, 400, 500}; printf("The given array is:\n"); for (int i = 0; i < 5; i++) { printf("%d ", arr[i]); } printf("\n"); printf("\nFor index 0, arr[0]: %d\n", arr[0]); printf("*(arr + 0) = %d\n", *(arr + 0)); printf("Address of arr[0] = %p\n\n", (void *)&arr[0]); printf("For index 1, arr[1]: %d\n", arr[1]); printf("*(arr + 1) = %d\n", *(arr + 1)); printf("Address of arr[1] = %p\n", (void *)&arr[1]); return 0; }
The output of the above code is as follows:
The given array is: 100 200 300 400 500 For index 0, arr[0]: 100 *(arr + 0) = 100 Address of arr[0] = 0x7ffc24dbfdf0 For index 1, arr[1]: 200 *(arr + 1) = 200 Address of arr[1] = 0x7ffc24dbfdf4
#include <iostream> using namespace std; int main() { int arr[] = {100, 200, 300, 400, 500}; cout << "The given array is: " << endl; for (int i = 0; i < 5; i++) { cout << arr[i] << " "; } cout << endl; cout << "\nFor index 0, arr[0]: " << arr[0] << endl; cout << "*(arr + 0) = " << *(arr + 0) << endl; cout << "Address of arr[0] = " << static_cast<void *>(&arr[0]) << endl << endl; cout << "For index 1, arr[1]: " << arr[1] << endl; cout << "*(arr + 1) = " << *(arr + 1) << endl; cout << "Address of arr[1] = " << static_cast<void *>(&arr[1]) << endl; return 0; }
The output of the above code is as follows:
The given array is: 100 200 300 400 500 For index 0, arr[0]: 100 *(arr + 0) = 100 Address of arr[0] = 0x7ffda70d26c0 For index 1, arr[1]: 200 *(arr + 1) = 200 Address of arr[1] = 0x7ffda70d26c4