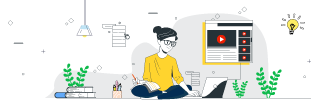
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Why is the Size of an Empty Class Not Zero in C++
The size of an empty class is not zero in C++ as it allocates one unique address to the object in the memory. The size can not be 0, because the two classes can not have the same memory address. So, the size of an empty class is 1 byte which does not hold any data, it is just for memory allocation.
In this article, we will see an example of checking the size of an object of an empty class in C++.
Demonstrating Size of an Empty Class
In this approach, we have two C++ classes. One class is an empty class while other is not an empty class. We have printed the size of both the classes as output. You can observe the size of empty and non-empty class in the example code given below.
Example
Here is an example demonstrating the size of both, empty and non-empty class:
#include <iostream> using namespace std; class Empty {}; class NotEmpty { public: int a = 4; }; int main() { cout << "Size of Empty class: " << sizeof(Empty) << endl; cout << "Size of NotEmpty class: " << sizeof(NotEmpty) << endl; return 0; }
The output of the above code is:
Size of Empty class: 1 Size of NotEmpty class: 4
Distinct Memory Allocation in Empty Classes
In this approach, we have two objects defined as a and b of the empty class MyClass. We have checked if the address of both objects is the same or not. Since there is a unique memory allocation it will print "not same".
Example
The following example demonstrates memory allocation of two objects in an empty class.
#include<iostream> using namespace std; class MyClass { }; int main() { MyClass a, b; if (&a == &b) cout <<"Same "<< endl; else cout <<"Not same "<< endl; }
The output of the above code is:
Not same
Dynamic Memory Allocation of Empty Classes
We have used the new keyword to create instances of two empty classes dynamically. The if/else statement checks if the pointers point to the same memory address or not.
Example
The following example demonstrates dynamic memory allocation of empty classes.
#include<iostream> using namespace std; class MyClass { }; int main() { MyClass *a = new MyClass(); MyClass *b = new MyClass(); if (a == b) cout <<"Same "<< endl; else cout <<"Not same "<< endl; }
The output of the above code is:
Not same