Building Powerful Telegram Bots with Telethon in Python
Last Updated :
19 Jun, 2024
Creating a Telegram bot can significantly enhance how you interact with users, automate tasks, and provide information quickly. Here, we’ll use the Telethon library in Python to build a powerful Telegram bot.
Creating Telegram Bot in Python
Prerequisites
- Telegram Account: We need a Telegram account to create and manage our bot.
- Telethon Library: We’ll use Telethon, a Python library for interacting with Telegram’s API.
Step 1: Install Telethon
pip install telethon
Step 2: Set Up the Telegram Bot
- Open Telegram and search for @BotFather.
- Start a chat and send the /newbot command.
- Follow the instructions to create a new bot. Then we’ll receive a bot token, which we need for authentication.
Step 3: Create a New Application
- Visit my.telegram.org and log in with our Telegram account.
- Go to the API Development Tools and create a new application.
- Note down the api_id and api_hash.
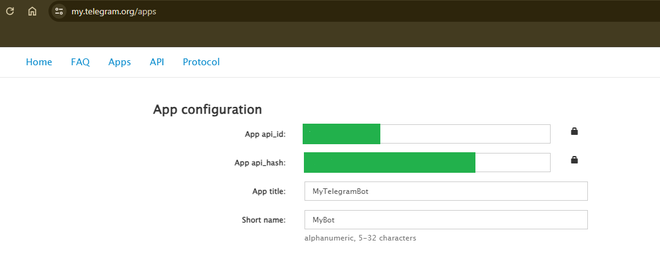
Note down the API Id & Hash key
Step 4: Write the Bot Script
- Logging Setup: Configures logging to help debug the bot.
- Telegram Client Initialization: Uses api_id, api_hash, and bot_token to create and start the Telegram client.
- Command Handlers:
- /start: Greets the user and offers assistance.
- /help: Provides a list of commands the bot can respond to.
- /info: Gives information about the bot.
- /echo <message>: Echoes back the message sent by the user.
- Keyword-based Responses: Responds to specific keywords and phrases with predefined messages.
- Default Response: Provides help information if the user’s message doesn’t match any known commands or keywords.
Python
import logging
from telethon import TelegramClient, events
# Setup logging
logging.basicConfig(level=logging.INFO)
# Your API ID and hash from my.telegram.org
api_id = "your_api_id"
api_hash = 'Your_hash_key'
bot_token = 'Your_bot_token' # Replace with your actual bot token
# Create the client and connect
client = TelegramClient('bot', api_id, api_hash).start(bot_token=bot_token)
# Handler for the /start command
@client.on(events.NewMessage(pattern='/start'))
async def start(event):
await event.respond('Hello! I am a Telethon bot. How can I assist you today?')
logging.info(f'Start command received from {event.sender_id}')
# Handler for the /help command
@client.on(events.NewMessage(pattern='/help'))
async def help(event):
help_text = (
"Here are the commands you can use:\n"
"/start - Start the bot\n"
"/help - Get help information\n"
"/info - Get information about the bot\n"
"/echo <message> - Echo back the message\n"
)
await event.respond(help_text)
logging.info(f'Help command received from {event.sender_id}')
# Handler for the /info command
@client.on(events.NewMessage(pattern='/info'))
async def info(event):
await event.respond('This bot is created using Telethon in Python. It can respond to various commands and messages.')
logging.info(f'Info command received from {event.sender_id}')
# Handler for the /echo command
@client.on(events.NewMessage(pattern='/echo (.+)'))
async def echo(event):
message = event.pattern_match.group(1)
await event.respond(f'Echo: {message}')
logging.info(f'Echo command received from {event.sender_id} with message: {message}')
# Keyword-based response handler
@client.on(events.NewMessage)
async def keyword_responder(event):
message = event.text.lower()
responses = {
'hello': 'Hi there! How can I help you today?',
'how are you': 'I am just a bot, but I am here to assist you!',
'what is your name': 'I am MyAwesomeBot, your friendly Telegram assistant.',
'bye': 'Goodbye! Have a great day!',
'time': 'I cannot tell the current time, but you can check your device!',
'date': 'I cannot provide the current date, but your device surely can!',
'weather': 'I cannot check the weather, but there are many apps that can help you with that!',
'thank you': 'You are welcome!',
'help me': 'Sure! What do you need help with?',
'good morning': 'Good morning! I hope you have a great day!',
'good night': 'Good night! Sweet dreams!',
'who created you': 'I was created by a developer using the Telethon library in Python.',
}
response = responses.get(message, None)
if response:
await event.respond(response)
else:
# Default response
default_response = (
"I didn't understand that command. Here are some commands you can try:\n"
"/start - Start the bot\n"
"/help - Get help information\n"
"/info - Get information about the bot\n"
"/echo <message> - Echo back the message\n"
)
await event.respond(default_response)
logging.info(f'Message received from {event.sender_id}: {event.text}')
# Start the client
client.start()
client.run_until_disconnected()
Step 5: Run the Bot
python telegram_bot.py
Output:
Conclusion
Building a Telegram bot with Telethon in Python is both rewarding and educational. This guide covers setting up the bot, handling various commands, and responding intelligently to user inputs. Using the Telethon library, the bot can efficiently manage interactions, provide information, and automate tasks. This foundation allows for further expansion of the bot’s features, integration with other services, and improved user engagement. Creating and customizing a Telegram bot offers valuable skills in API usage, Python programming, and real-time interaction management, paving the way for more advanced projects in the future.
Please Login to comment...